print(map(str,range (5)))
时间: 2023-12-03 17:04:21 浏览: 23
这段代码会返回一个 map 对象,对象中包含了将 0 到 4 这 5 个整数转化为字符串后的结果。如果想要输出这些字符串,可以使用 list 函数将 map 对象转换为列表,然后再使用 print 函数输出。如下所示:
```
print(list(map(str, range(5))))
```
输出结果为:
```
['0', '1', '2', '3', '4']
```
相关问题
map(str,range(10))
这是一个 Python 中的语法,它将一个字符串和一个范围内的数字序列映射起来,返回一个迭代器对象。具体实现可以参考以下代码:
```python
result = map(str, range(10))
print(list(result))
```
输出结果为:['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']
在python中 x[:3]=map(str,range(5))
这行代码的含义是,将0到4的整数转换为字符串后,将前3个字符串赋值给x的前3个元素。
例如:
```python
x = ['a', 'b', 'c', 'd', 'e']
x[:3] = map(str, range(5))
print(x) # ['0', '1', '2', 'd', 'e']
```
在上述例子中,range(5)生成的是0到4的整数,map(str, range(5))将这些整数转换为字符串。然后,通过切片操作x[:3],将前3个元素替换为这些字符串,因此最终的结果是['0', '1', '2', 'd', 'e']。
相关推荐
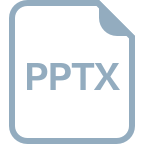
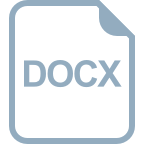
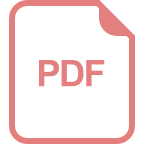













