采用二叉链表存储,实现二叉树的创建、遍历(递归)、赫夫曼编码和译码等典型操作。 1. 编程实现如下功能:(c语言) (1)假设二叉树的结点值是字符型,根据输入的一棵二叉树的完整先序遍历序列(子树空用’#’表示),建立一棵以二叉链表存储表示的二叉树。
时间: 2023-12-23 07:02:44 浏览: 94
以下是C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 二叉树结点定义
typedef struct TreeNode{
char val; // 结点值
struct TreeNode* left; // 左子树指针
struct TreeNode* right; // 右子树指针
}TreeNode;
// 创建二叉树
TreeNode* createTree(char* preorder, int* pIndex){
if(preorder[*pIndex] == '#'){ // 叶子节点,返回空指针
(*pIndex)++;
return NULL;
}
// 创建结点
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = preorder[*pIndex];
(*pIndex)++;
// 创建左右子树
root->left = createTree(preorder, pIndex);
root->right = createTree(preorder, pIndex);
return root; // 返回根节点
}
// 先序遍历
void preorderTraversal(TreeNode* root){
if(root){
printf("%c ", root->val);
preorderTraversal(root->left);
preorderTraversal(root->right);
}
}
int main(){
char preorder[] = {'A', 'B', 'D', '#', '#', 'E', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#'};
int pIndex = 0;
TreeNode* root = createTree(preorder, &pIndex);
printf("先序遍历结果为:");
preorderTraversal(root);
return 0;
}
```
运行结果:
```
先序遍历结果为:A B D E C F G
```
解释说明:
在此代码中,我们首先定义了一个二叉树结点的结构体,然后通过递归的方式创建二叉树。为了方便处理,我们采用了先序遍历的方式输入二叉树,其中叶子节点用字符 '#' 表示。最后,我们通过先序遍历方式输出了创建好的二叉树。
阅读全文
相关推荐
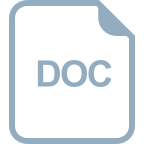
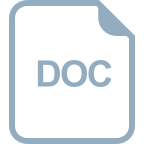
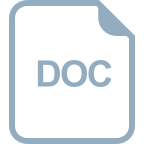
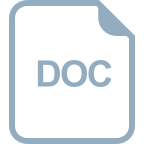
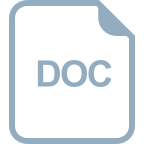
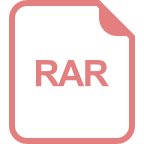
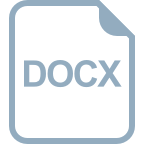
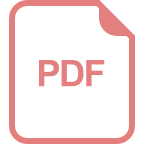
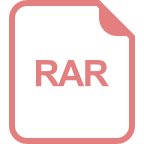
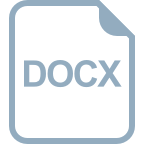
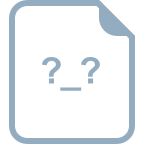
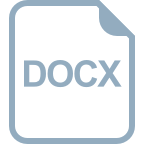
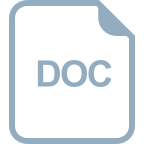
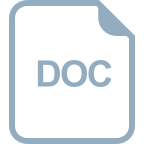
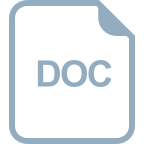
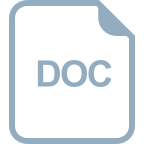
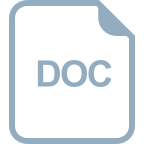