本关任务:编写一个函数: char *ReplaceString(char * str, char * s1, char * s2); 该函数用s2替换str中的s1,函数返回替换后的字符串的指针。 题目中已经给出main函数,main函数会调用ReplaceString函数进行测试,依次输入字符串str、s1和s2,最后输出替换后字符串。
时间: 2024-03-16 11:46:26 浏览: 27
好的,下面是实现ReplaceString函数的代码示例:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
char* ReplaceString(char* str, const char* s1, const char* s2) {
char* p = strstr(str, s1);
if (p == NULL) {
return str;
}
int len1 = strlen(s1);
int len2 = strlen(s2);
int len = strlen(str) - len1 + len2 + 1;
char* newstr = (char*)malloc(len * sizeof(char));
memset(newstr, 0, len);
strncpy(newstr, str, p - str);
strcat(newstr, s2);
strcat(newstr, p + len1);
return newstr;
}
int main() {
char str[100], s1[100], s2[100];
scanf("%s%s%s", str, s1, s2);
printf("%s\n", ReplaceString(str, s1, s2));
return 0;
}
```
在ReplaceString函数中,我们首先使用strstr函数在str字符串中找到s1的位置,如果没有找到,则直接返回str字符串的地址。如果找到了s1的位置,我们计算出替换后字符串的长度,并使用malloc函数动态分配内存来存储替换后的字符串。
接着,我们使用strncpy函数将str字符串中s1之前的部分复制到newstr中,然后使用strcat函数将s2字符串连接到newstr的末尾,最后使用strcat函数将str字符串中s1之后的部分连接到newstr的末尾。最后返回newstr字符串的地址。
在main函数中,我们依次输入字符串str、s1和s2,然后调用ReplaceString函数将s1替换为s2,并输出替换后的字符串。
相关推荐
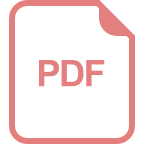
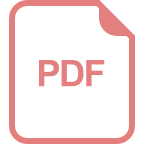
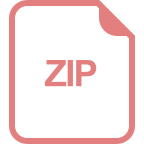










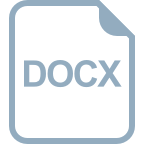
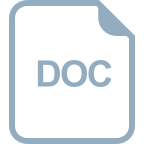
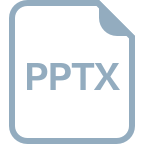
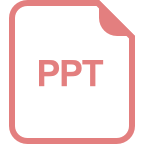
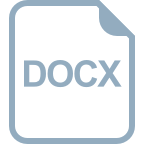
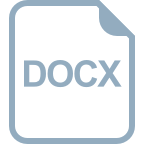