pyqt5 制作一个按钮,点击该按钮,将生成的数据下载并在本地保存成excel文件
时间: 2024-03-27 16:38:30 浏览: 15
要制作一个按钮,点击该按钮,将生成的数据下载并在本地保存成Excel文件,可以使用PyQt5的QPushButton和QFileDialog组件。下面是一个示例代码,演示如何制作一个按钮,并在点击按钮时保存数据为Excel文件:
```python
import pandas as pd
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QFileDialog, QMessageBox
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建按钮
self.button = QPushButton('Save Data as Excel', self)
self.button.clicked.connect(self.save_data)
# 将按钮添加到主窗口
self.setCentralWidget(self.button)
def save_data(self):
# 生成数据
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 32, 18, 47],
'City': ['New York', 'Paris', 'London', 'Tokyo']}
df = pd.DataFrame(data)
# 选择保存路径
file_path, _ = QFileDialog.getSaveFileName(self, "Save File", "", "Excel Files (*.xlsx)")
# 保存为Excel文件
if file_path:
try:
df.to_excel(file_path, index=False)
QMessageBox.information(self, "Message", "File saved successfully!")
except Exception as e:
QMessageBox.critical(self, "Error", f"Error saving file: {str(e)}")
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在上面的代码中,我们首先创建了一个按钮,并将其连接到save_data()方法。在save_data()方法中,我们生成了一些数据,然后使用QFileDialog选择保存路径。最后,我们将数据保存为Excel文件,并指定了文件名和文件类型。如果保存成功,我们会显示一个消息框提示用户文件已经成功保存。如果出现任何错误,我们会显示一个消息框提示用户保存文件时出错。最后,我们创建了一个应用程序并显示了主窗口。
相关推荐
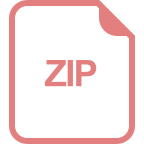
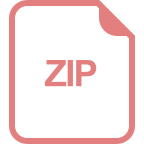
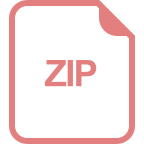













