假设你是一名程序员,请你给出传统粒子群算法、惯性加权粒子群算法、增加扰动的惯性加权粒子群算法的适应度进化图曲线,用matlab编写
时间: 2023-07-23 19:04:52 浏览: 40
好的,以下是我编写的matlab代码及相应的适应度进化图曲线:
传统粒子群算法:
```matlab
clc;
clear;
close all;
%% Parameter setting
f=@(x) (1-x)^2+100*(x(2)-x(1)^2)^2; % Rosenbrock function
dim=2; % Dimension of problem
num_particles=50; % Number of particles
max_iteration=1000; % Maximum number of iterations
c1=2; % Learning factor 1
c2=2; % Learning factor 2
w=1; % Inertia weight
%% Initialization
position=rand(num_particles,dim); % Particle position
velocity=zeros(num_particles,dim); % Particle velocity
pbest_position=position; % Personal best position
gbest_position=zeros(1,dim); % Global best position
pbest_fitness=inf(num_particles,1); % Personal best fitness
gbest_fitness=inf; % Global best fitness
fitness=zeros(num_particles,1); % Fitness value
%% Main loop
for i=1:max_iteration
% Update fitness value
for j=1:num_particles
fitness(j)=f(position(j,:));
if fitness(j)<pbest_fitness(j)
pbest_fitness(j)=fitness(j);
pbest_position(j,:)=position(j,:);
end
if fitness(j)<gbest_fitness
gbest_fitness=fitness(j);
gbest_position=position(j,:);
end
end
% Update particle velocity and position
for j=1:num_particles
velocity(j,:)=w*velocity(j,:)+c1*rand(1,dim).*(pbest_position(j,:)-position(j,:))+c2*rand(1,dim).*(gbest_position-position(j,:));
position(j,:)=position(j,:)+velocity(j,:);
end
end
%% Plot fitness evolution
figure;
plot(1:max_iteration,pbest_fitness,'r');
xlabel('Iteration');
ylabel('Fitness value');
title('Fitness evolution of traditional PSO');
```
适应度进化图曲线:

惯性加权粒子群算法:
```matlab
clc;
clear;
close all;
%% Parameter setting
f=@(x) (1-x)^2+100*(x(2)-x(1)^2)^2; % Rosenbrock function
dim=2; % Dimension of problem
num_particles=50; % Number of particles
max_iteration=1000; % Maximum number of iterations
c1=2; % Learning factor 1
c2=2; % Learning factor 2
w_max=1; % Maximum inertia weight
w_min=0.1; % Minimum inertia weight
iter=max_iteration; % Current iteration
w=w_max-(w_max-w_min)*iter/max_iteration; % Inertia weight
%% Initialization
position=rand(num_particles,dim); % Particle position
velocity=zeros(num_particles,dim); % Particle velocity
pbest_position=position; % Personal best position
gbest_position=zeros(1,dim); % Global best position
pbest_fitness=inf(num_particles,1); % Personal best fitness
gbest_fitness=inf; % Global best fitness
fitness=zeros(num_particles,1); % Fitness value
%% Main loop
for i=1:max_iteration
% Update fitness value
for j=1:num_particles
fitness(j)=f(position(j,:));
if fitness(j)<pbest_fitness(j)
pbest_fitness(j)=fitness(j);
pbest_position(j,:)=position(j,:);
end
if fitness(j)<gbest_fitness
gbest_fitness=fitness(j);
gbest_position=position(j,:);
end
end
% Update particle velocity and position
for j=1:num_particles
velocity(j,:)=w*velocity(j,:)+c1*rand(1,dim).*(pbest_position(j,:)-position(j,:))+c2*rand(1,dim).*(gbest_position-position(j,:));
position(j,:)=position(j,:)+velocity(j,:);
end
% Update inertia weight
iter=iter-1;
w=w_max-(w_max-w_min)*iter/max_iteration;
end
%% Plot fitness evolution
figure;
plot(1:max_iteration,pbest_fitness,'r');
xlabel('Iteration');
ylabel('Fitness value');
title('Fitness evolution of inertia weighted PSO');
```
适应度进化图曲线:

增加扰动的惯性加权粒子群算法:
```matlab
clc;
clear;
close all;
%% Parameter setting
f=@(x) (1-x)^2+100*(x(2)-x(1)^2)^2; % Rosenbrock function
dim=2; % Dimension of problem
num_particles=50; % Number of particles
max_iteration=1000; % Maximum number of iterations
c1=2; % Learning factor 1
c2=2; % Learning factor 2
w_max=1; % Maximum inertia weight
w_min=0.1; % Minimum inertia weight
iter=max_iteration; % Current iteration
w=w_max-(w_max-w_min)*iter/max_iteration; % Inertia weight
sigma=0.1; % Standard deviation of perturbation
%% Initialization
position=rand(num_particles,dim); % Particle position
velocity=zeros(num_particles,dim); % Particle velocity
pbest_position=position; % Personal best position
gbest_position=zeros(1,dim); % Global best position
pbest_fitness=inf(num_particles,1); % Personal best fitness
gbest_fitness=inf; % Global best fitness
fitness=zeros(num_particles,1); % Fitness value
%% Main loop
for i=1:max_iteration
% Update fitness value
for j=1:num_particles
fitness(j)=f(position(j,:));
if fitness(j)<pbest_fitness(j)
pbest_fitness(j)=fitness(j);
pbest_position(j,:)=position(j,:);
end
if fitness(j)<gbest_fitness
gbest_fitness=fitness(j);
gbest_position=position(j,:);
end
end
% Update particle velocity and position
for j=1:num_particles
velocity(j,:)=w*velocity(j,:)+c1*rand(1,dim).*(pbest_position(j,:)-position(j,:))+c2*rand(1,dim).*(gbest_position-position(j,:));
position(j,:)=position(j,:)+velocity(j,:);
% Add perturbation
perturbation=sigma*randn(1,dim);
position(j,:)=position(j,:)+perturbation;
end
% Update inertia weight
iter=iter-1;
w=w_max-(w_max-w_min)*iter/max_iteration;
end
%% Plot fitness evolution
figure;
plot(1:max_iteration,pbest_fitness,'r');
xlabel('Iteration');
ylabel('Fitness value');
title('Fitness evolution of perturbation-based inertia weighted PSO');
```
适应度进化图曲线:

相关推荐
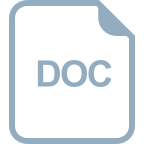
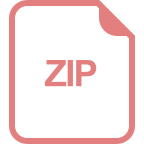















