C++输人10个学生5门课的成绩,能够按任意一门课程的成绩,对学生信息进行排序,并将排序结果写入到文件students.txt中,程序启动时,能自动从文件students.txt中读取学生的信息
时间: 2024-02-27 20:58:02 浏览: 24
可以使用文件流来读取和写入文件,具体操作如下:
```cpp
#include <iostream>
#include <fstream>
#include <algorithm>
using namespace std;
struct Student {
string name;
int scores[5];
double average;
};
bool cmp(Student a, Student b) {
// 根据第二门课程成绩进行排序
return a.scores[1] > b.scores[1];
}
int main() {
const int N = 10, M = 5;
Student students[N];
// 从文件中读取学生信息
ifstream infile("students.txt");
for (int i = 0; i < N; i++) {
infile >> students[i].name;
for (int j = 0; j < M; j++) {
infile >> students[i].scores[j];
}
students[i].average = accumulate(students[i].scores, students[i].scores + M, 0) / (double)M;
}
infile.close();
// 根据第二门课程成绩进行排序
sort(students, students + N, cmp);
// 将排序后的结果写入到文件中
ofstream outfile("students.txt");
for (int i = 0; i < N; i++) {
outfile << students[i].name << " ";
for (int j = 0; j < M; j++) {
outfile << students[i].scores[j] << " ";
}
outfile << "平均分:" << students[i].average << endl;
}
outfile.close();
return 0;
}
```
注:以上代码仅是示例代码,实际使用时需要根据具体需求进行修改。另外,在写入文件时需要注意,如果不想覆盖原有的内容,可以使用`ofstream`的`ios::app`参数,表示以追加的方式打开文件,如下:
```cpp
ofstream outfile("students.txt", ios::app);
```
相关推荐
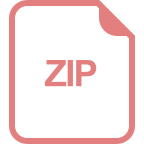
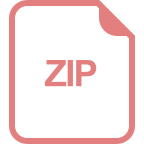
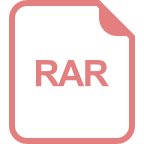
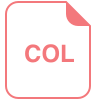
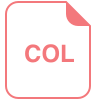
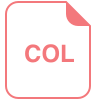
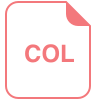
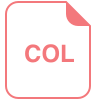









