class Node(Generic[T]): def __init__(self, state: T, parent: Optional[Node], cost: float = 0, heuristic: float = 0) -> None: self.state: T = state self.parent: Optional[Node] = parent self.cost: float = cost self.heuristic = heuristic def __lt__(self, other): return (self.cost + self.heuristic) < (other.cost + other.heuristic) def node_to_path2(node: Node[T]) -> List[T]: path: List[T] = [node.state] while node.parent is not None: node = node.parent path.append(node.state) # print(node.state) path.reverse() return path class PriorityQueue(Generic[T]): def __init__(self): self._container: List[T] = [] @property def empty(self): return not self._container def push(self, item: T): heappush(self._container, item) def pop(self): return heappop(self._container) def __repr__(self): return repr(self._container)这段代码每行什么意思帮我加上注释
时间: 2024-03-31 20:31:47 浏览: 92
# 定义节点类,泛型类型为 T
class Node(Generic[T]):
def __init__(self, state: T, parent: Optional[Node], cost: float = 0, heuristic: float = 0) -> None:
self.state: T = state # 节点的状态
self.parent: Optional[Node] = parent # 节点的父节点
self.cost: float = cost # 起点到该节点的路径代价
self.heuristic = heuristic # 该节点到终点的估计代价(启发函数值)
def __lt__(self, other): # 重载小于号,用于优先队列的比较
return (self.cost + self.heuristic) < (other.cost + other.heuristic)
# 定义将节点转化为路径的函数,泛型类型为 T
def node_to_path2(node: Node[T]) -> List[T]:
path: List[T] = [node.state] # 路径,初始化为节点的状态
while node.parent is not None: # 如果节点有父节点
node = node.parent # 将当前节点更新为其父节点
path.append(node.state) # 将父节点的状态加入路径中
path.reverse() # 将路径反转,使其按照起点到终点的顺序排列
return path
# 定义优先队列类,泛型类型为 T
class PriorityQueue(Generic[T]):
def __init__(self):
self._container: List[T] = [] # 容器,用于存储元素
@property
def empty(self): # 判断队列是否为空
return not self._container
def push(self, item: T): # 将元素加入队列
heappush(self._container, item)
def pop(self): # 从队列中取出最小元素
return heappop(self._container)
def __repr__(self): # 重载输出函数,输出队列中的元素
return repr(self._container)
阅读全文
相关推荐
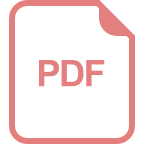
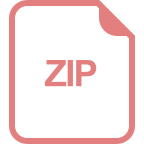
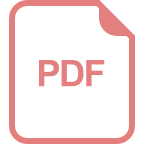

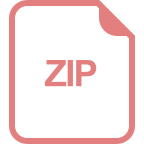
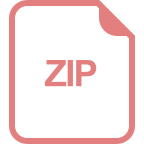
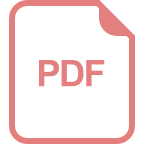
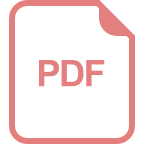
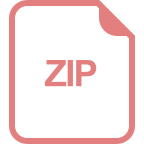
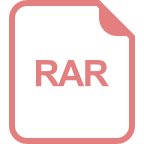
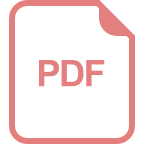
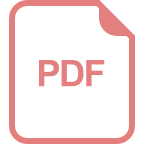
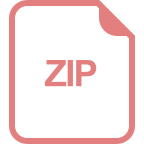
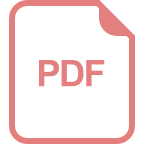
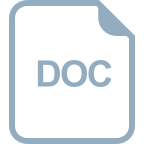
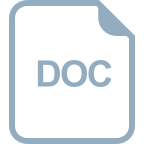
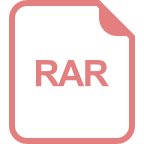