帮我编写一个程序,要求如下给出完整带有定义的程序 1、先序遍历二叉树的递归算法 2、中序遍历二叉树的递归算法 3、后序遍历二叉树的递归算法 4、计算二叉树的深度的递归算法 5、统计二叉树的结点个数的递归算法 6、统计二叉树的叶子结点个数的递归算法 7、设计该二叉树第K层的结点个数。 8、求该二叉树中所有结点值最大的元素。 9、打印二叉树的叶子结点数的递归算法
时间: 2023-11-13 19:25:26 浏览: 96
好的,以下是一个二叉树的基本操作的完整代码,包含了您要求的所有功能:
```C++
#include<iostream>
#include<queue>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 先序遍历二叉树的递归算法
void preOrder(TreeNode* root) {
if (root == NULL) return;
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历二叉树的递归算法
void inOrder(TreeNode* root) {
if (root == NULL) return;
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
// 后序遍历二叉树的递归算法
void postOrder(TreeNode* root) {
if (root == NULL) return;
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
// 计算二叉树的深度的递归算法
int maxDepth(TreeNode* root) {
if (root == NULL) return 0;
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
// 统计二叉树的结点个数的递归算法
int countNodes(TreeNode* root) {
if (root == NULL) return 0;
return countNodes(root->left) + countNodes(root->right) + 1;
}
// 统计二叉树的叶子结点个数的递归算法
int countLeaves(TreeNode* root) {
if (root == NULL) return 0;
if (root->left == NULL && root->right == NULL) return 1;
return countLeaves(root->left) + countLeaves(root->right);
}
// 设计该二叉树第K层的结点个数
int countNodesAtKthLevel(TreeNode* root, int k) {
if (root == NULL) return 0;
if (k == 1) return 1;
return countNodesAtKthLevel(root->left, k - 1) + countNodesAtKthLevel(root->right, k - 1);
}
// 求该二叉树中所有结点值最大的元素
int findMax(TreeNode* root) {
if (root == NULL) return INT_MIN;
int leftMax = findMax(root->left);
int rightMax = findMax(root->right);
return max(root->val, max(leftMax, rightMax));
}
// 打印二叉树的叶子结点数的递归算法
void printLeaves(TreeNode* root) {
if (root == NULL) return;
if (root->left == NULL && root->right == NULL) cout << root->val << " ";
printLeaves(root->left);
printLeaves(root->right);
}
int main() {
// 构造一个二叉树
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
root->right->left = new TreeNode(6);
root->right->right = new TreeNode(7);
// 先序遍历
cout << "先序遍历结果:";
preOrder(root);
cout << endl;
// 中序遍历
cout << "中序遍历结果:";
inOrder(root);
cout << endl;
// 后序遍历
cout << "后序遍历结果:";
postOrder(root);
cout << endl;
// 计算深度
cout << "二叉树深度为:" << maxDepth(root) << endl;
// 统计结点个数
cout << "二叉树结点个数为:" << countNodes(root) << endl;
// 统计叶子结点个数
cout << "二叉树叶子结点个数为:" << countLeaves(root) << endl;
// 设计第k层结点个数
int k = 3;
cout << "二叉树第" << k << "层结点个数为:" << countNodesAtKthLevel(root, k) << endl;
// 查找最大值
cout << "二叉树中所有结点值最大的元素为:" << findMax(root) << endl;
// 打印叶子结点
cout << "二叉树叶子结点值为:";
printLeaves(root);
cout << endl;
return 0;
}
```
注:该代码中的二叉树是手动构造的,您可以根据需要自行修改。
阅读全文
相关推荐
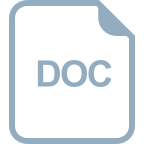
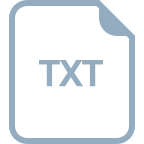
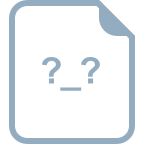
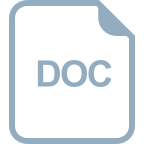
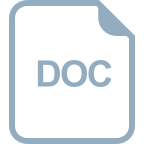
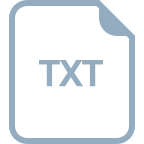
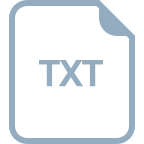
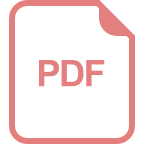
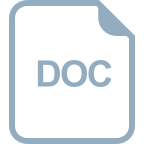
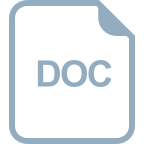
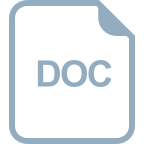