加减乘除计算器c语言if
时间: 2024-11-12 14:40:09 浏览: 33
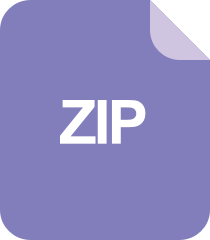
caculatorv1.1_简加减乘除计算器_
在C语言中,你可以使用if语句来编写一个简单的加减乘除计算器程序。首先,你需要定义一个函数,比如`calculator`,接收两个操作数和一个运算符作为输入。然后,根据运算符的不同,使用if-else if结构来判断并执行相应的计算:
```c
#include <stdio.h>
// 定义计算函数
int calculator(int a, int b, char op) {
int result;
// 判断运算符并执行相应操作
if (op == '+') { // 加法
result = a + b;
} else if (op == '-') { // 减法
result = a - b;
} else if (op == '*') { // 乘法
result = a * b;
} else if (op == '/') { // 除法
if (b != 0) { // 防止除以零错误
result = a / b;
} else {
printf("Error! Division by zero is not allowed.\n");
return 0; // 或者抛出异常
}
} else {
printf("Invalid operator.\n");
return 0; // 对于未知或非基本运算符处理
}
return result;
}
int main() {
int num1, num2;
char operation;
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
printf("Enter the operator (+, -, *, /): ");
scanf(" %c", &operation); // 需要额外的空格防止scanf读取换行符
int result = calculator(num1, num2, operation);
if (result) {
printf("Result: %d\n", result);
}
return 0;
}
```
在这个示例中,用户会被提示输入两个数字和一个运算符,然后程序会根据用户的输入执行对应的计算。注意,这个程序假设用户输入的是正确的运算符,并且处理了除以零的情况。
阅读全文
相关推荐
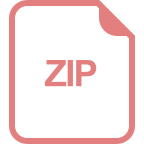
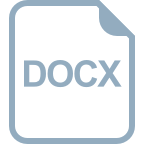















