@Override public void paint(Graphics g) { if(iBuffer == null) { iBuffer = createImage(this.getSize().width,this.getSize().height); gBuffer = iBuffer.getGraphics(); } for(int i=0;i<11;i++) { for(int j=0;j<11;j++) { gBuffer.drawImage(mapimg[tempmap[level][i][j]-1], j*32, i*32, 32, 32, this); } }
时间: 2024-03-18 09:45:40 浏览: 12
这段代码是一个自定义的面板类中的方法,它是绘制游戏画面的方法。该方法使用了 @Override 注解,表示它重写了父类的 paint 方法。在方法中,首先检查缓冲图像 iBuffer 是否为 null,如果是,则创建一个和面板大小相同的缓冲图像,并且获取它的 Graphics 对象。接着,使用两个嵌套的 for 循环来遍历地图中的每一个元素,根据元素的值来绘制相应的图像。具体来说,它使用 tempmap 数组中当前关卡的地图信息,获取地图元素值,并且从图像数组 mapimg 中获取相应的图像。然后,使用 Graphics 对象 gBuffer 的 drawImage 方法将图像绘制在缓冲图像上,并且根据元素在地图中的位置来确定绘制位置和大小。最终,整个缓冲图像将会被绘制在屏幕上,完成游戏画面的绘制。
相关问题
public class JavaMain { public static void main(String[] args) { new MyWindow(); } } class MyWindow extends JFrame implements MouseListener, MouseMotionListener, ActionListener { //定义两个点 private Point oldPoint = null,newPoint = null; public MyWindow () { this.setSize(600,600); addMouseListener(this); addMouseMotionListener(this); this.setVisible(true); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } //画图 public void paint(Graphics g) { Graphics2D g2 = (Graphics2D) g; g2.setStroke(new BasicStroke(10.0f)); g2.setColor(Color.red); if(oldPoint!=null&&newPoint!=null) { g2.drawLine(oldPoint.x, oldPoint.y, newPoint.x, newPoint.y); oldPoint=newPoint; newPoint=null;//置为空,为了迎接下一个点的到来 } } //鼠标拖动事件 @Override public void mouseDragged(MouseEvent e) { if(oldPoint==null) { oldPoint=e.getPoint(); }else { newPoint=e.getPoint(); repaint();//生成新的点,通知系统去更新绘图 } } @Override public void mouseMoved(MouseEvent e) {} //鼠标单击事件 @Override public void mouseClicked(MouseEvent e) {} @Override public void mouseEntered(MouseEvent e) {} @Override public void mouseExited(MouseEvent e) {} @Override public void mousePressed(MouseEvent e) {} //鼠标释放事件,清空工作 @Override public void mouseReleased(MouseEvent e) { newPoint=null; oldPoint=null; } }设置清除画布按钮
您可以在 `MyWindow` 类中添加一个按钮,并为其添加一个 `ActionListener`,在按钮被点击时清除画布。代码示例如下:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class MyWindow extends JFrame implements MouseListener, MouseMotionListener, ActionListener {
private Point oldPoint = null, newPoint = null;
private JButton clearButton; // 添加一个清除按钮
public MyWindow() {
this.setSize(600, 600);
addMouseListener(this);
addMouseMotionListener(this);
// 初始化清除按钮
clearButton = new JButton("清除画布");
clearButton.addActionListener(this);
JPanel buttonPanel = new JPanel();
buttonPanel.add(clearButton);
add(buttonPanel, BorderLayout.SOUTH);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setStroke(new BasicStroke(10.0f));
g2.setColor(Color.red);
if (oldPoint != null && newPoint != null) {
g2.drawLine(oldPoint.x, oldPoint.y, newPoint.x, newPoint.y);
oldPoint = newPoint;
newPoint = null;
}
}
@Override
public void mouseDragged(MouseEvent e) {
if (oldPoint == null) {
oldPoint = e.getPoint();
} else {
newPoint = e.getPoint();
repaint();
}
}
@Override
public void mouseMoved(MouseEvent e) {}
@Override
public void mouseClicked(MouseEvent e) {}
@Override
public void mouseEntered(MouseEvent e) {}
@Override
public void mouseExited(MouseEvent e) {}
@Override
public void mousePressed(MouseEvent e) {}
@Override
public void mouseReleased(MouseEvent e) {
newPoint = null;
oldPoint = null;
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == clearButton) {
Graphics g = getGraphics();
g.setColor(getBackground());
g.fillRect(0, 0, getWidth(), getHeight());
}
}
}
```
在上面的代码中,我们添加了一个 `clearButton` 按钮,并在其上添加了一个 `ActionListener`。当按钮被点击时,会调用 `actionPerformed` 方法清除画布。在 `actionPerformed` 方法中,我们获取了 `MyWindow` 的 `Graphics` 对象,并使用 `fillRect` 方法覆盖整个画布。这样就达到了清除画布的效果。
@Override public void onBindViewHolder(@NonNull ShowListAdapter.ViewHolder holder, int position) { }编写弹窗的内容
好的,以下是一个简单的弹窗示例,你可以在 `onBindViewHolder` 方法中调用:
```java
@Override
public void onBindViewHolder(@NonNull ShowListAdapter.ViewHolder holder, int position) {
// 点击 item 弹出弹窗
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// 创建弹窗
AlertDialog.Builder builder = new AlertDialog.Builder(mContext);
builder.setTitle("弹窗标题");
builder.setMessage("弹窗内容");
// 设置确定按钮
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
// 点击确定按钮后的逻辑处理
}
});
// 设置取消按钮
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
// 点击取消按钮后的逻辑处理
}
});
// 显示弹窗
AlertDialog alertDialog = builder.create();
alertDialog.show();
}
});
}
```
以上代码中,我们创建了一个 `AlertDialog.Builder` 对象,设置了弹窗的标题和内容,以及确定和取消按钮的逻辑处理。最后调用 `create` 方法创建 `AlertDialog` 对象并显示出来。你可以根据自己的需要自定义弹窗的样式和逻辑。
相关推荐
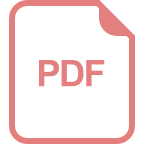













