uniapp点击刷新组件
时间: 2023-09-30 14:11:25 浏览: 72
UniApp中提供了下拉刷新组件`uni-refresher`,可以用于实现下拉刷新功能。
使用方法如下:
1. 在需要添加下拉刷新功能的页面中添加`uni-refresher`组件:
```html
<template>
<view>
<uni-refresher @refresh="onRefresh">
<!-- 下拉刷新内容区域 -->
</uni-refresher>
</view>
</template>
```
2. 在`methods`中添加`onRefresh`方法处理下拉刷新事件:
```javascript
methods: {
onRefresh() {
// 处理下拉刷新事件
}
}
```
3. 在`onRefresh`方法中编写刷新逻辑,完成刷新后调用`uni.stopPullDownRefresh()`方法停止下拉刷新动画:
```javascript
methods: {
onRefresh() {
// 处理下拉刷新事件
setTimeout(() => {
// 模拟刷新完成
uni.stopPullDownRefresh()
}, 1000)
}
}
```
参考文档:[uni-refresher](https://uniapp.dcloud.io/component/uni-refresher)
相关问题
uniapp下拉刷新组件代码
以下是一个示例的uniapp下拉刷新组件代码:
```html
<template>
<view>
<view class="refresh-text">{{refreshText}}</view>
<scroll-view :style="{height:scrollViewHeight + 'px'}" scroll-y="true" @scrolltoupper="onScrollToUpper" @scroll="onScroll">
<view class="list">
<view class="list-item" v-for="(item, index) in list" :key="index">
{{item}}
</view>
</view>
</scroll-view>
</view>
</template>
<script>
export default {
data() {
return {
list: [], // 数据列表
page: 1, // 当前页码
isLoading: false, // 是否正在加载数据
refreshText: '下拉刷新', // 刷新文本
startScrollTop: 0, // 滚动条开始位置
scrollViewHeight: 0, // 滚动区域高度
};
},
methods: {
// 下拉刷新
onScrollToUpper() {
if (this.isLoading) {
return;
}
this.isLoading = true;
this.refreshText = '正在刷新...';
// 模拟加载数据
setTimeout(() => {
this.list = ['刷新数据1', '刷新数据2', '刷新数据3'];
this.page = 1;
this.isLoading = false;
this.refreshText = '下拉刷新';
}, 2000);
},
// 滚动事件
onScroll(e) {
if (this.isLoading) {
return;
}
const scrollTop = e.detail.scrollTop;
const scrollViewHeight = e.detail.scrollHeight;
const windowHeight = uni.getSystemInfoSync().windowHeight;
if (scrollTop < this.startScrollTop && scrollTop <= 0) {
// 向上滚动并且滚动到顶部,触发下拉刷新
this.onScrollToUpper();
} else if (scrollViewHeight - (scrollTop + windowHeight) <= 10) {
// 滚动到底部,触发加载更多
this.onLoadMore();
}
this.startScrollTop = scrollTop;
this.scrollViewHeight = scrollViewHeight;
},
// 加载更多
onLoadMore() {
if (this.isLoading) {
return;
}
this.isLoading = true;
// 模拟加载数据
setTimeout(() => {
this.list = [...this.list, `加载更多数据${this.page + 1}`, `加载更多数据${this.page + 2}`, `加载更多数据${this.page + 3}`];
this.page++;
this.isLoading = false;
}, 2000);
},
},
};
</script>
```
这个示例包含一个下拉刷新组件和一个滚动加载更多组件,可以在下拉到顶部时触发下拉刷新,滚动到底部时触发加载更多。
uniapp刷新子组件
在uniapp中,可以使用$refs来获取子组件的实例,然后调用子组件的方法来刷新子组件。
下面是一个示例代码:
// 父组件
<template>
<div>
<child ref="child"></child>
<button @click="refreshChild">刷新子组件</button>
</div>
</template>
<script>
import child from './components/child.vue'
export default {
components: {
child
},
methods: {
refreshChild() {
this.$refs.child.refresh()
}
}
}
</script>
// 子组件
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello World'
}
},
methods: {
refresh() {
// 刷新操作
}
}
}
</script>
在父组件中,我们使用$refs来获取子组件的实例,并调用子组件的refresh方法来刷新子组件。在子组件中,我们定义了一个refresh方法,用于执行刷新操作。
阅读全文
相关推荐
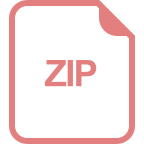
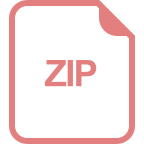













