2)定义学生类Student,包含学号、姓名、年龄和若干门课的成绩,要求: 键盘输入信息对一个学生对象初始化: 求学生的平均成绩。 输出所有信息。c++
时间: 2024-02-24 10:57:40 浏览: 133
好的,根据你的要求,我来编写一个学生类的程序,如下所示:
```c++
#include <iostream>
#include <string>
using namespace std;
const int NUM_COURSES = 3; // 假设每个学生只有三门课程
class Student {
private:
string id; // 学号
string name; // 姓名
int age; // 年龄
double scores[NUM_COURSES]; // 课程成绩
public:
// 构造函数
Student() {
id = "";
name = "";
age = 0;
for (int i = 0; i < NUM_COURSES; i++) {
scores[i] = 0;
}
}
// 从键盘输入学生信息
void input() {
cout << "请输入学生信息:" << endl;
cout << "学号:";
cin >> id;
cout << "姓名:";
cin >> name;
cout << "年龄:";
cin >> age;
cout << "各门课的成绩:" << endl;
for (int i = 0; i < NUM_COURSES; i++) {
cout << "第" << i+1 << "门课:";
cin >> scores[i];
}
}
// 计算平均成绩
double getAverageScore() {
double sum = 0;
for (int i = 0; i < NUM_COURSES; i++) {
sum += scores[i];
}
return sum / NUM_COURSES;
}
// 输出学生信息
void print() {
cout << "学生信息如下:" << endl;
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
cout << "各门课成绩:";
for (int i = 0; i < NUM_COURSES; i++) {
cout << scores[i] << " ";
}
cout << endl;
cout << "平均成绩:" << getAverageScore() << endl;
}
};
int main() {
Student stu;
stu.input();
stu.print();
return 0;
}
```
程序中,我们定义了一个 `Student` 类,并且在类中封装了学号、姓名、年龄和若干门课程的成绩。通过 `input()` 方法从键盘输入学生信息,通过 `getAverageScore()` 方法计算平均成绩,通过 `print()` 方法输出学生信息。在 `main()` 函数中,我们创建一个 `Student` 对象 `stu`,并且调用 `input()` 和 `print()` 方法对学生信息进行输入和输出。
阅读全文
相关推荐
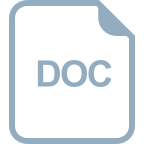
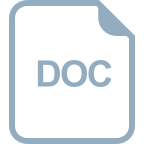
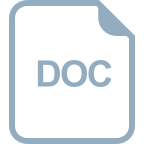
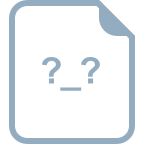
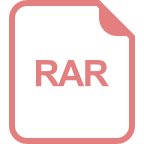
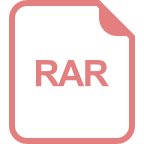
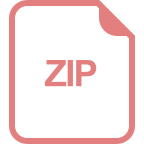
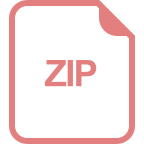
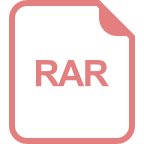
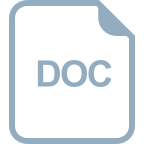
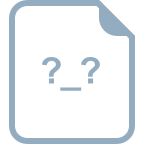
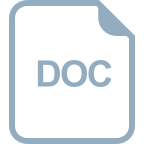
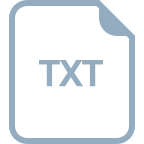
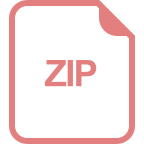
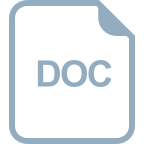
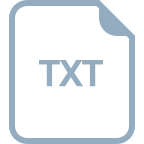
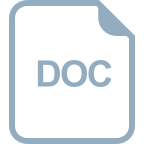
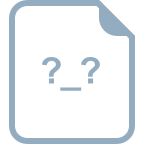
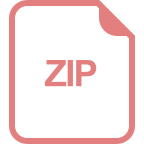