c++重载函数复数计算器
时间: 2023-06-14 21:06:01 浏览: 134
下面是一个简单的C++复数计算器,其中重载了加法、减法、乘法和除法运算符,可以进行复数的加减乘除运算。
```c++
#include <iostream>
using namespace std;
class Complex
{
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(const Complex& other) const
{
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const
{
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const
{
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const
{
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator);
}
friend ostream& operator<<(ostream& os, const Complex& c)
{
os << c.real << "+" << c.imag << "i";
return os;
}
};
int main()
{
Complex a(2, 3);
Complex b(4, 5);
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a + b = " << a + b << endl;
cout << "a - b = " << a - b << endl;
cout << "a * b = " << a * b << endl;
cout << "a / b = " << a / b << endl;
return 0;
}
```
在上面的代码中,我们定义了一个名为Complex的类,它有两个私有成员变量real和imag,表示实部和虚部。我们定义了一个构造函数,用于初始化实部和虚部。
然后我们重载了加法、减法、乘法和除法运算符,使得我们可以像操作普通数字一样操作复数。在重载运算符时,我们返回一个新的Complex对象,其中包含了运算结果。
最后,我们定义了一个友元函数operator<<,用于将Complex对象输出到流中,以便我们可以在控制台中查看运算结果。
阅读全文
相关推荐
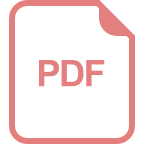
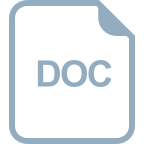
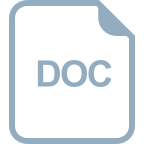
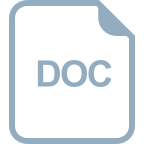
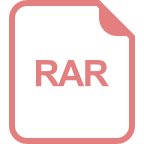
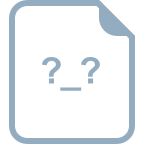
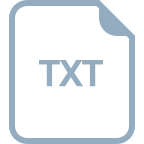
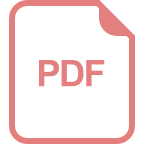
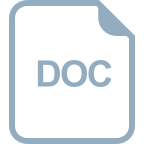
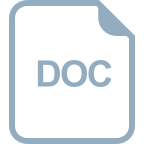
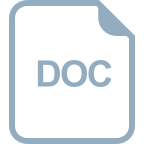
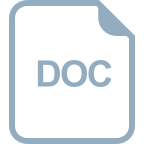
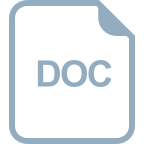
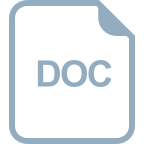

