onSubmit() { let params = { name: this.sizeForm.name, beginDate: this.sizeForm.beginDate, endDate: this.sizeForm.endDate, pageNum: 1, pageSize: 10 }; axios.get('http://localhost:8085/LoginAndRegister/fill/selectFill', params).then(response => { console.log(response.data); this.tableData = response.data.rows; this.total = response.data.total; }).catch(error => { console.log(error); }); }, 这段代码哪里不对’
时间: 2024-04-29 20:20:40 浏览: 21
这段代码中,axios.get()方法的第二个参数应该是一个配置对象,而不是一个简单的参数对象。应该将params对象放入配置对象的params属性中,如下所示:
```
axios.get('http://localhost:8085/LoginAndRegister/fill/selectFill', {
params: {
name: this.sizeForm.name,
beginDate: this.sizeForm.beginDate,
endDate: this.sizeForm.endDate,
pageNum: 1,
pageSize: 10
}
}).then(response => {
console.log(response.data);
this.tableData = response.data.rows;
this.total = response.data.total;
}).catch(error => {
console.log(error);
});
```
另外,在使用axios进行网络请求时,需要注意跨域问题。如果接口地址和前端页面不在同一个域名下,需要在后端进行CORS配置或使用代理来解决跨域问题。
相关问题
// 分页 handleSizeChange(val) { this.pageSize = val this.handleNodeClick() }, handleCurrentChange(val) { this.page = val this.handleNodeClick() }, async getTreeListList() { const { data } = await businessTreeApi() this.departlist = JSON.parse(data.data) }, // 右侧上部分信息 async handleNodeClick(type) { console.log(type) if (type) { this.id = type.id } const List = (await businessApi(this.id)).data.data // this.datalist.agentId = type.id ? type.id : id // console.log(this.datalist) // const {data}= await courierPagingApi(this.datalist) // console.log(data); console.log(List) if (List.type === 1) { this.form.type = '一级转运中心' } else if (List.type === 2) { this.form.type = '二级转运中心' } else this.form.type = '营业部' this.form.number = List.id // v-model 数字 // this.form.type=List.type==3?'营业部':'', // 类型 this.form.name = List.name // 名字 this.form.region = List.province.name // 省 this.form.city = List.city.name // 城市 this.form.county = List.county.name // 县区 this.form.address = List.address // 详细地址 this.form.people = List.managerName // 负责人 this.form.phone = List.phone // 电话 this.form.longitude = List.longitude // 经度 this.form.latitude = List.latitude // 维度 // ------------------------------------ let obj = { page: this.page, pageSize: this.pageSize, agencyId: this.id } // 页码 const { data } = await getUserPageApi(obj) this.total = data.data.counts console.log(data.data) this.tableData = data.data.items this.total = Number(data.data.counts) // 下拉框数据 const res = await editAreasApi() console.log(res) this.economize = res.data.data if (this.value) { const ress = await editAreasApi(this.value) console.log(ress) } }, onSubmit() { this.isOk = !this.isOk } // 编辑数据 }
这段代码是关于一个树形结构的页面分页展示和编辑数据的处理,其中包括以下几个方法:
1. `handleSizeChange(val)`:处理每页展示数据量的变化,并调用`handleNodeClick()`方法重新渲染数据。
2. `handleCurrentChange(val)`:处理当前页码的变化,并调用`handleNodeClick()`方法重新渲染数据。
3. `getTreeListList()`:获取树形结构的数据,并将其解析为JSON格式。
4. `handleNodeClick(type)`:处理节点的点击事件,并根据节点类型展示对应的数据。同时,根据当前节点的ID,获取该节点下的用户数据,并展示在页面中。另外,还会获取下拉框数据。
5. `onSubmit()`:处理编辑数据的提交事件。
总体来说,这段代码实现了一个树形结构的页面展示和编辑数据的功能。
优化代码 methods: { onSubmit(values) { console.log("submit", values); if(values.username === this.$username && values.password === this.$password) { localStorage.setItem("Token", false) this.$router.push({path: '/'}) } } }
这段代码可以进行以下优化:
1. 将 `values` 对象中的属性解构出来,以便更方便地获取 `username` 和 `password` 属性的值。
2. 将对 `$username` 和 `$password` 的访问改为对组件实例中的 `username` 和 `password` 属性的访问,以避免使用 `$` 符号。
3. 将 `localStorage.setItem` 方法的值改为 `true`,以表示用户已经通过身份验证,并将该值的键名改为一个常量,以避免硬编码。
4. 在调用 `$router.push` 方法时,使用命名路由(name)而不是路径(path),以提高代码的可读性。
优化后的代码如下所示:
```
const TOKEN_KEY = "Token";
methods: {
onSubmit({ username, password }) {
console.log("submit", { username, password });
if (username === this.username && password === this.password) {
localStorage.setItem(TOKEN_KEY, true);
this.$router.push({ name: "home" });
}
}
}
```
其中,假设在路由配置中有一个名为 `home` 的路由项。
相关推荐
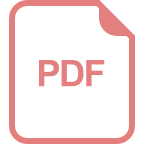
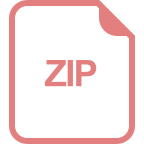
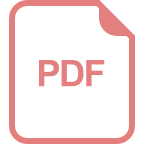













