我想在wpf中自定义路由事件,要求当超过一个值时,小灯开始闪烁
时间: 2023-07-16 19:11:37 浏览: 41
首先,我们需要定义一个路由事件。在WPF中,我们可以使用`RoutedEvent`类来定义一个路由事件。下面是一个示例:
```csharp
public class MyCustomControl : Control
{
// 定义路由事件
public static readonly RoutedEvent MyCustomEvent = EventManager.RegisterRoutedEvent(
"MyCustom", // 事件名
RoutingStrategy.Bubble, // 事件路由策略
typeof(RoutedEventHandler), // 事件处理委托类型
typeof(MyCustomControl) // 定义事件的所有者类型
);
// 添加路由事件处理程序
public event RoutedEventHandler MyCustom
{
add { AddHandler(MyCustomEvent, value); }
remove { RemoveHandler(MyCustomEvent, value); }
}
// 触发路由事件
private void RaiseMyCustomEvent()
{
RoutedEventArgs args = new RoutedEventArgs(MyCustomEvent);
RaiseEvent(args);
}
}
```
接下来,我们需要在`MyCustomControl`类中添加一个依赖属性来存储阈值,并在属性值发生变化时触发路由事件。下面是一个示例:
```csharp
public class MyCustomControl : Control
{
// 定义路由事件和依赖属性
public static readonly RoutedEvent MyCustomEvent = EventManager.RegisterRoutedEvent(...);
public static readonly DependencyProperty ThresholdProperty = DependencyProperty.Register(
"Threshold", typeof(double), typeof(MyCustomControl),
new PropertyMetadata(0.0, OnThresholdChanged)
);
// 添加依赖属性的包装器
public double Threshold
{
get { return (double)GetValue(ThresholdProperty); }
set { SetValue(ThresholdProperty, value); }
}
// 属性值变化时触发路由事件
private static void OnThresholdChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
MyCustomControl control = d as MyCustomControl;
double newValue = (double)e.NewValue;
if (newValue > control.Threshold)
{
control.RaiseMyCustomEvent();
}
}
// ...
}
```
最后,我们可以在XAML中使用`MyCustomControl`并添加路由事件处理程序来控制小灯的闪烁。下面是一个示例:
```xaml
<Window x:Class="WpfApp1.MainWindow"
xmlns:local="clr-namespace:WpfApp1"
...>
<Grid>
<local:MyCustomControl Threshold="50" MyCustom="MyCustomControl_MyCustom"/>
<Ellipse x:Name="Light" Fill="Green" Width="20" Height="20"/>
</Grid>
</Window>
```
```csharp
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void MyCustomControl_MyCustom(object sender, RoutedEventArgs e)
{
Light.Fill = Brushes.Red;
Storyboard blinkStoryboard = new Storyboard();
DoubleAnimation opacityAnimation = new DoubleAnimation
{
From = 1,
To = 0,
Duration = TimeSpan.FromSeconds(0.5),
RepeatBehavior = RepeatBehavior.Forever,
AutoReverse = true
};
Storyboard.SetTarget(opacityAnimation, Light);
Storyboard.SetTargetProperty(opacityAnimation, new PropertyPath(Ellipse.OpacityProperty));
blinkStoryboard.Children.Add(opacityAnimation);
blinkStoryboard.Begin();
}
}
```
在`MyCustomControl_MyCustom`事件处理程序中,我们可以控制小灯的颜色和闪烁动画。当`Threshold`属性的值超过50时,路由事件将被触发,并且小灯将开始闪烁。
相关推荐
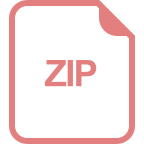














