假设有这么个字符串集合:{“abc","123","234","abc","123"},使用map<const char*,int>m记录各个字符串出现的次数
时间: 2023-09-01 20:12:44 浏览: 69
,输出m中每个元素的字符串和出现次数。
很高兴为您解答。以下是代码片段:
```C++
#include <iostream>
#include <map>
#include <string>
int main()
{
std::string arr[] = {"abc", "123", "234", "abc", "123"}; // 字符串数组
std::map<const char*, int> m; // 定义映射表
for (const auto& str : arr) // 遍历字符串数组
{
++m[str.c_str()]; // 计数器+1
}
// 输出每个元素的字符串和出现次数
for (const auto& p : m)
{
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
```
输出结果如下:
```
123: 2
234: 1
abc: 2
```
希望能帮到您!
相关问题
class InformationUI { public: /*视角0第一视角1第三视角*/ int viewAngleEnum; /*球标图*/ int bubble; /*是否英制螺牙*/ int isEnglishSystem; /*是否添加研磨符号*/ int isLap; /*球标图大小*/ double bubbleSize; /*图纸路径*/ std::string drawSheetPath; /*客户标准*/ std::string customer; /*被选中零件标记*/ std::string selLable; /*投影视图0主视图1右视图2前视图3左视图4后视图5正三轴*/ int view[6]; /*公共属性and属性值*/ std::map<std::string, std::string> commonAttrAndVal; /*临时部件*/ static std::string tempPartName; };如何这个类换为二进制格式,并将其保存到文件中
可以使用序列化技术将该类转换为二进制格式,并将其保存到文件中。以下是示例代码:
```cpp
#include <fstream>
#include <iostream>
#include <cstring>
#include <map>
#include <sstream>
class InformationUI {
public:
/*视角0第一视角1第三视角*/
int viewAngleEnum;
/*球标图*/
int bubble;
/*是否英制螺牙*/
int isEnglishSystem;
/*是否添加研磨符号*/
int isLap;
/*球标图大小*/
double bubbleSize;
/*图纸路径*/
std::string drawSheetPath;
/*客户标准*/
std::string customer;
/*被选中零件标记*/
std::string selLable;
/*投影视图0主视图1右视图2前视图3左视图4后视图5正三轴*/
int view[6];
/*公共属性and属性值*/
std::map<std::string, std::string> commonAttrAndVal;
/*临时部件*/
static std::string tempPartName;
// 序列化成二进制格式
std::string serialize() {
std::stringstream ss;
// 写入基本类型变量
ss.write(reinterpret_cast<char*>(&viewAngleEnum), sizeof(viewAngleEnum));
ss.write(reinterpret_cast<char*>(&bubble), sizeof(bubble));
ss.write(reinterpret_cast<char*>(&isEnglishSystem), sizeof(isEnglishSystem));
ss.write(reinterpret_cast<char*>(&isLap), sizeof(isLap));
ss.write(reinterpret_cast<char*>(&bubbleSize), sizeof(bubbleSize));
// 写入字符串
int strSize = drawSheetPath.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(drawSheetPath.c_str(), strSize);
strSize = customer.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(customer.c_str(), strSize);
strSize = selLable.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(selLable.c_str(), strSize);
// 写入整型数组
ss.write(reinterpret_cast<char*>(view), sizeof(view));
// 写入map
strSize = commonAttrAndVal.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
for (auto it = commonAttrAndVal.begin(); it != commonAttrAndVal.end(); ++it) {
strSize = it->first.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(it->first.c_str(), strSize);
strSize = it->second.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(it->second.c_str(), strSize);
}
// 写入静态变量
strSize = tempPartName.size();
ss.write(reinterpret_cast<char*>(&strSize), sizeof(strSize));
ss.write(tempPartName.c_str(), strSize);
return ss.str();
}
// 从二进制格式反序列化
void deserialize(const std::string& data) {
std::stringstream ss(data);
// 读取基本类型变量
ss.read(reinterpret_cast<char*>(&viewAngleEnum), sizeof(viewAngleEnum));
ss.read(reinterpret_cast<char*>(&bubble), sizeof(bubble));
ss.read(reinterpret_cast<char*>(&isEnglishSystem), sizeof(isEnglishSystem));
ss.read(reinterpret_cast<char*>(&isLap), sizeof(isLap));
ss.read(reinterpret_cast<char*>(&bubbleSize), sizeof(bubbleSize));
// 读取字符串
int strSize;
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
drawSheetPath.resize(strSize);
ss.read(&drawSheetPath[0], strSize);
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
customer.resize(strSize);
ss.read(&customer[0], strSize);
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
selLable.resize(strSize);
ss.read(&selLable[0], strSize);
// 读取整型数组
ss.read(reinterpret_cast<char*>(view), sizeof(view));
// 读取map
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
commonAttrAndVal.clear();
for (int i = 0; i < strSize; ++i) {
std::string key, value;
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
key.resize(strSize);
ss.read(&key[0], strSize);
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
value.resize(strSize);
ss.read(&value[0], strSize);
commonAttrAndVal[key] = value;
}
// 读取静态变量
ss.read(reinterpret_cast<char*>(&strSize), sizeof(strSize));
tempPartName.resize(strSize);
ss.read(&tempPartName[0], strSize);
}
};
// 保存到文件
void saveToFile(const std::string& filename, const InformationUI& info) {
// 序列化
std::string data = info.serialize();
// 写入文件
std::ofstream fout(filename, std::ios::binary);
fout.write(data.c_str(), data.size());
}
// 从文件读取
InformationUI readFromFile(const std::string& filename) {
// 读取文件
std::ifstream fin(filename, std::ios::binary);
std::string data((std::istreambuf_iterator<char>(fin)), std::istreambuf_iterator<char>());
// 反序列化
InformationUI info;
info.deserialize(data);
return info;
}
int main() {
InformationUI info;
info.viewAngleEnum = 1;
info.bubble = 2;
info.isEnglishSystem = 1;
info.isLap = 0;
info.bubbleSize = 3.14;
info.drawSheetPath = "test.dwg";
info.customer = "ABC";
info.selLable = "DEF";
info.view[0] = 0;
info.view[1] = 1;
info.commonAttrAndVal["attr1"] = "value1";
info.commonAttrAndVal["attr2"] = "value2";
InformationUI::tempPartName = "temp";
saveToFile("test.bin", info);
InformationUI info2 = readFromFile("test.bin");
std::cout << info2.viewAngleEnum << std::endl;
std::cout << info2.bubble << std::endl;
std::cout << info2.isEnglishSystem << std::endl;
std::cout << info2.isLap << std::endl;
std::cout << info2.bubbleSize << std::endl;
std::cout << info2.drawSheetPath << std::endl;
std::cout << info2.customer << std::endl;
std::cout << info2.selLable << std::endl;
std::cout << info2.view[0] << std::endl;
std::cout << info2.view[1] << std::endl;
std::cout << info2.commonAttrAndVal["attr1"] << std::endl;
std::cout << info2.commonAttrAndVal["attr2"] << std::endl;
std::cout << InformationUI::tempPartName << std::endl;
return 0;
}
```
在上面的示例中,我们使用`serialize()`函数将`InformationUI`类序列化为二进制格式,在`deserialize()`函数中将二进制格式反序列化为`InformationUI`类。`saveToFile()`函数将序列化后的数据保存到文件中,`readFromFile()`函数从文件中读取数据并反序列化为`InformationUI`类。
使用c++读入一个英文文本文件,英文中有字母、数字、其它字符,无汉字,文件有多行(程序要能处理至少4行文字,附件中有参考素材)。 2.编写函数实现:统计字母的出现频度,并显示结果。(大小写算同一字母) 3.编写函数实现:统计其中单词的个数,并显示结果。(单词个数以WORD统计作为参考,不一定100%相同。) 4.编写函数实现:找出最长的单词,并显示单词及其长度。 5.编写函数实现:统计出现的整数,存入一维数组,并显示结果。 (1) 比如文件中有两行: "abc 123 W 6 www 9." "teacher 10 student 7." (2) 分析得到5个数:123、6、9、10、7。 (3) 定义数组int a[1000] (4) a[0]=123,a[1]=6,a[2]=9,a[3]=10,a[4]=7。 注:可以利用下标或字符型指针处理字符串。
以下是一个基础版的实现,可以根据需求进行修改:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <map>
#include <vector>
#include <sstream>
using namespace std;
// 统计字母的出现频度
void countLetters(const string& text, map<char, int>& freq) {
for (char c : text) {
if (isalpha(c)) { // 只统计字母
c = tolower(c); // 转换为小写字母
freq[c]++;
}
}
}
// 统计单词的个数
int countWords(const string& text) {
stringstream ss(text);
string word;
int count = 0;
while (ss >> word) {
count++;
}
return count;
}
// 找出最长的单词
string findLongestWord(const string& text) {
stringstream ss(text);
string word, longestWord;
int maxLength = 0;
while (ss >> word) {
int length = word.length();
if (length > maxLength) {
maxLength = length;
longestWord = word;
}
}
return longestWord;
}
// 统计出现的整数
void countIntegers(const string& text, vector<int>& integers) {
stringstream ss(text);
string word;
while (ss >> word) {
if (isdigit(word[0])) { // 判断是否为数字
int num = stoi(word);
integers.push_back(num);
}
}
}
int main() {
ifstream infile("input.txt");
string line;
map<char, int> freq;
int wordCount = 0;
string longestWord;
int maxLength = 0;
vector<int> integers;
while (getline(infile, line)) {
countLetters(line, freq);
wordCount += countWords(line);
string word = findLongestWord(line);
int length = word.length();
if (length > maxLength) {
maxLength = length;
longestWord = word;
}
countIntegers(line, integers);
}
// 输出字母频度
cout << "Letter Frequency:" << endl;
for (auto p : freq) {
cout << p.first << ": " << p.second << endl;
}
// 输出单词个数
cout << "Word Count: " << wordCount << endl;
// 输出最长的单词及其长度
cout << "Longest Word: " << longestWord << ", Length: " << maxLength << endl;
// 输出整数
cout << "Integers: ";
for (int i : integers) {
cout << i << " ";
}
cout << endl;
return 0;
}
```
其中,`countLetters`函数使用`map`来统计字母的出现频度,`countWords`函数使用`stringstream`来分离单词并统计个数,`findLongestWord`函数遍历单词并记录最长单词,`countIntegers`函数使用`stringstream`来判断数字并存入向量中。在`main`函数中遍历每一行文本,调用上述函数来实现各项功能。
阅读全文
相关推荐
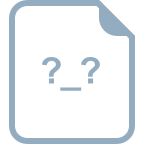
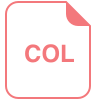
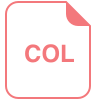
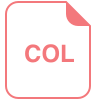
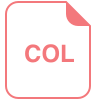
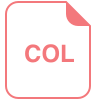





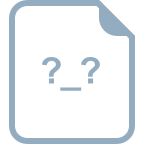
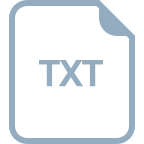
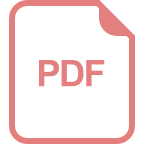