springboot拦截字段上的注解并获取注解对应的对象值
时间: 2023-08-06 10:06:59 浏览: 51
在Spring Boot中,可以使用拦截器和注解来实现拦截字段上的注解并获取注解对应的对象值。
首先,我们需要自定义一个注解,例如:
```
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface MyAnnotation {
String value();
}
```
这个注解定义了一个value属性,用来存储注解对应的对象值。
然后,我们需要定义一个拦截器,在拦截器中获取字段上的注解,并将注解对应的对象值存储到请求上下文中,例如:
```
public class MyInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
Field[] fields = handlerMethod.getMethod().getDeclaringClass().getDeclaredFields();
for (Field field : fields) {
if (field.isAnnotationPresent(MyAnnotation.class)) {
MyAnnotation annotation = field.getAnnotation(MyAnnotation.class);
String value = annotation.value();
request.setAttribute(field.getName(), value);
}
}
}
return true;
}
}
```
在这个拦截器中,我们通过反射获取到当前处理请求的方法所在的类,然后遍历这个类中定义的所有字段,如果这个字段上有我们定义的注解,就从注解中获取对象值,并将这个值存储到请求上下文中,以便后续的处理程序可以使用这个值。
最后,我们需要在Spring Boot中注册这个拦截器,例如:
```
@Configuration
public class InterceptorConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new MyInterceptor());
}
}
```
在这个配置类中,我们实现了WebMvcConfigurer接口,并覆盖了它的addInterceptors方法,将我们定义的拦截器注册到Spring Boot中。
这样,当请求到达处理程序时,我们就可以从请求上下文中获取到字段上的注解对应的对象值了。
相关推荐
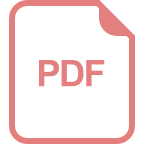
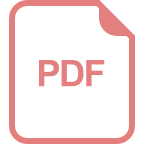
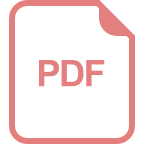














