k均值聚类算法 matlab
时间: 2023-06-30 11:18:38 浏览: 68
k均值聚类算法是一种常见的无监督学习算法,可以用于将一组数据分成k个不同的簇。在Matlab中,可以使用自带的kmeans函数实现该算法。下面是一个简单的Matlab代码示例:
```
% 生成一组随机数据
data = rand(100,2);
% 将数据分成3个簇
k = 3;
% 使用kmeans函数进行聚类
[idx, C] = kmeans(data, k);
% 可视化聚类结果
scatter(data(:,1), data(:,2), 10, idx, 'filled');
hold on;
scatter(C(:,1), C(:,2), 50, 'k', 'filled');
```
在上述示例中,我们首先生成了一组随机数据,然后使用kmeans函数将数据分成了3个簇。最后,我们将聚类结果可视化出来,其中不同的颜色表示不同的簇,黑色的点表示每个簇的中心点。
相关问题
k均值聚类算法matlab
k均值聚类算法是一种常见的聚类算法,可以使用Matlab进行实现。以下是一份简单的Matlab代码实现k均值聚类算法:
```matlab
% 输入数据
data = [1, 1; 1.5, 2; 3, 4; 5, 7; 3.5, 5; 4.5, 5; 3.5, 4.5];
K = 2; % 聚类数
[m, n] = size(data); % 数据维度和数据点个数
% 初始化聚类中心
center = zeros(K, n);
index = randperm(m, K);
for i = 1:K
center(i, :) = data(index(i), :);
end
% 迭代求解聚类中心
max_iter = 100; % 最大迭代次数
iter = 0;
while iter < max_iter
iter = iter + 1;
% 分配样本到聚类中心
cluster = cell(K, 1);
for i = 1:m
dist = zeros(K, 1);
for j = 1:K
dist(j) = norm(data(i, :) - center(j, :));
end
[~, idx] = min(dist);
cluster{idx} = [cluster{idx}; data(i, :)];
end
% 更新聚类中心
for i = 1:K
center(i, :) = mean(cluster{i}, 1);
end
end
% 可视化结果
figure;
hold on;
colors = ['r', 'g', 'b', 'c', 'm', 'y', 'k'];
for i = 1:K
scatter(cluster{i}(:, 1), cluster{i}(:, 2), 36, colors(i), 'filled');
end
scatter(center(:, 1), center(:, 2), 100, 'k', 'filled', 'd');
title('k-means clustering');
```
代码中的输入数据是一个二维数组,每一行代表一个数据点,K表示聚类数。该代码使用随机选择的方式初始化聚类中心,然后通过迭代不断更新聚类中心和分配样本到聚类中心,最终得到聚类结果并可视化。
kmeans均值聚类算法matlab
### 回答1:
在 MATLAB 中,可以使用 `kmeans` 函数实现 K 均值聚类算法。以下是一个简单的示例:
假设有一个包含 100 个样本的数据集,每个样本有两个特征。我们想将这些样本分成 3 类。
```matlab
% 生成样本数据
data = rand(100, 2);
% 使用 kmeans 函数进行聚类
[idx, centroids] = kmeans(data, 3);
% 绘制聚类结果
scatter(data(:, 1), data(:, 2), [], idx);
hold on;
scatter(centroids(:, 1), centroids(:, 2), [], 'black', 'filled');
```
在上面的代码中,`data` 变量是一个 100x2 的矩阵,表示样本数据。`kmeans` 函数的第一个参数是数据矩阵,第二个参数是要分成的类别数。函数的返回值 `idx` 是一个包含每个样本所属类别的向量,`centroids` 是一个包含每个类别的中心点坐标的矩阵。
最后,我们使用 `scatter` 函数绘制了聚类结果。每个样本的颜色表示它所属的类别,黑色的点表示每个类别的中心点。
### 回答2:
K均值聚类算法是一种无监督学习的算法,用于将数据集划分为K个簇,使得簇内的样本点尽可能地相似,而不同簇之间的样本点差异较大。在Matlab中,可以使用以下步骤实现K均值聚类算法:
1. 初始化聚类中心:随机选择K个样本点作为初始的聚类中心。
2. 分配样本点到簇:遍历所有样本点,计算每个样本点与各个聚类中心的距离,将样本点分配到距离最近的簇中。
3. 更新聚类中心:根据新分配的样本点,重新计算每个簇的聚类中心。
4. 重复步骤2和3,直到达到终止条件,如达到最大迭代次数或聚类中心不再发生变化。
最常用的距离度量是欧氏距离,但在Matlab中也可以选择其他距离度量方式。K均值聚类算法的性能会受到初始聚类中心和K值的选择影响,因此可以通过多次运行算法来选择最佳的初始聚类中心和K值。
在Matlab中,可以使用函数kmeans来实现K均值聚类算法。此函数需要输入待聚类的数据集和聚类数量K,输出结果是每个样本点所属的簇编号和最终的聚类中心。可以根据聚类结果进行后续的数据分析和可视化展示。
总之,K均值聚类算法是一种常用的无监督学习算法,能够将数据集划分为K个簇。在Matlab中,可以使用kmeans函数实现K均值聚类算法,并根据具体需求选择合适的聚类中心和K值。
### 回答3:
K-means均值聚类算法是一种常用的聚类方法,它在MATLAB中有相应的实现。
K-means算法的基本思想是根据数据点之间的距离,将它们划分到K个不同的簇中。首先,需要确定簇的数量K。然后,选取K个随机的数据点作为初始的簇中心点。接下来的迭代过程中,将每个数据点分配到离其最近的簇中心点所在的簇,然后根据分配结果重新计算簇中心点。重复这个过程,直到簇中心点的位置不再改变或达到最大迭代次数为止。
在MATLAB中,可以使用`kmeans`函数来实现K-means算法。这个函数的用法如下:
[idx, C] = kmeans(X, K)
其中,X是一个n×d的数据矩阵,n表示数据点的数量,d表示每个数据点的维度;K表示簇的数量。该函数返回两个输出:
- idx是一个n×1的向量,表示每个数据点所属的簇的索引。
- C是一个K×d的矩阵,表示每个簇的中心点的位置。
除了这些基本的输入和输出之外,还可以通过其他参数来进一步控制K-means算法的行为,例如设置最大迭代次数、初始的簇中心点、重复运行的次数等。
总而言之,K-means均值聚类算法是一种在MATLAB中实现的聚类方法,可以帮助我们将数据分成不同的簇。使用`kmeans`函数可以方便地进行K-means聚类,并获得每个数据点所属的簇的索引以及簇的中心点位置。
相关推荐
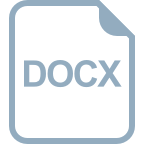
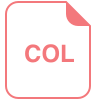



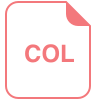







