办公考勤系统python代码实现
时间: 2023-08-21 12:04:48 浏览: 153
办公考勤系统是一个较为复杂的项目,需要涉及到多个方面的知识,如数据库设计、Web开发、前端设计等。以下是一个简单的Python代码实现考勤系统的示例,仅供参考:
1. 首先,安装并导入Flask框架
```python
pip install Flask
from flask import Flask, request, render_template
```
2. 设计数据库结构
```python
import sqlite3
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS users
(id integer primary key autoincrement, name text, email text unique, password text)''')
c.execute('''CREATE TABLE IF NOT EXISTS attendance
(id integer primary key autoincrement, user_id integer, date text, status text)''')
conn.commit()
conn.close()
```
3. 实现用户注册与登录
```python
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
name = request.form['name']
email = request.form['email']
password = request.form['password']
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute("INSERT INTO users (name, email, password) VALUES (?,?,?)", (name, email, password))
conn.commit()
conn.close()
return "User registered successfully!"
return render_template('register.html')
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
email = request.form['email']
password = request.form['password']
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute("SELECT * FROM users WHERE email=? AND password=?", (email, password))
user = c.fetchone()
conn.close()
if user:
session['user_id'] = user[0]
return redirect(url_for('home'))
else:
return "Invalid email or password"
return render_template('login.html')
```
4. 实现考勤打卡功能
```python
@app.route('/clock_in', methods=['GET', 'POST'])
def clock_in():
if request.method == 'POST':
user_id = session['user_id']
date = datetime.now().strftime("%Y-%m-%d")
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute("SELECT * FROM attendance WHERE user_id=? AND date=?", (user_id, date))
attendance = c.fetchone()
if attendance:
return "You have already clocked in today"
else:
c.execute("INSERT INTO attendance (user_id, date, status) VALUES (?,?,?)", (user_id, date, 'Clocked In'))
conn.commit()
conn.close()
return "Clock in success!"
return render_template('clock_in.html')
@app.route('/clock_out', methods=['GET', 'POST'])
def clock_out():
if request.method == 'POST':
user_id = session['user_id']
date = datetime.now().strftime("%Y-%m-%d")
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute("SELECT * FROM attendance WHERE user_id=? AND date=?", (user_id, date))
attendance = c.fetchone()
if not attendance:
return "You haven't clocked in yet"
elif attendance[3] == 'Clocked Out':
return "You have already clocked out today"
else:
c.execute("UPDATE attendance SET status=? WHERE id=?", ('Clocked Out', attendance[0]))
conn.commit()
conn.close()
return "Clock out success!"
return render_template('clock_out.html')
```
5. 实现考勤记录查询功能
```python
@app.route('/attendance', methods=['GET', 'POST'])
def attendance():
user_id = session['user_id']
conn = sqlite3.connect('attendance_system.db')
c = conn.cursor()
c.execute("SELECT * FROM attendance WHERE user_id=?", (user_id,))
records = c.fetchall()
conn.close()
return render_template('attendance.html', records=records)
```
以上是一个简单的Python代码实现考勤系统的示例,仅供参考。实际项目中,需要根据需求进行更加细致的设计和实现。
阅读全文
相关推荐
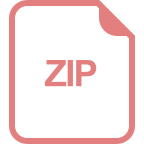


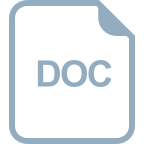

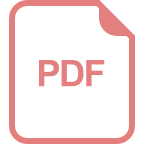
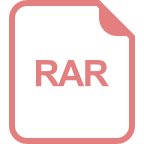
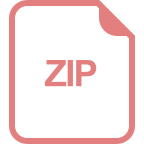
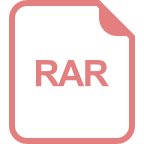
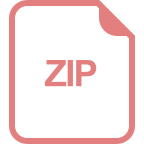
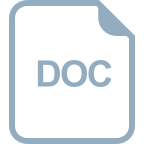
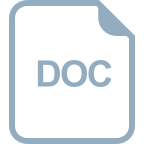
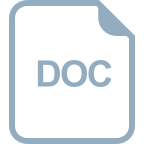
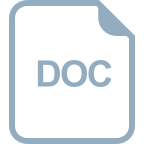
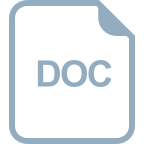
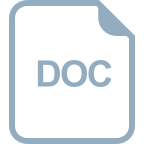
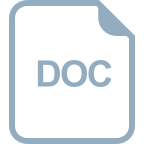