帮我写一个目标检测的程序,用python语言
时间: 2023-08-21 19:07:34 浏览: 105
好的,目标检测是计算机视觉中的一个重要任务,可以通过使用深度学习模型来实现。下面是一个基本的目标检测程序示例,使用Python语言和OpenCV库:
```python
import cv2
# 加载图像
img = cv2.imread('path/to/image.jpg')
# 加载预训练模型
model = cv2.dnn.readNetFromDarknet('path/to/yolov3.cfg', 'path/to/yolov3.weights')
# 获取输出层
output_layer_names = model.getLayerNames()
output_layers = [output_layer_names[i[0] - 1] for i in model.getUnconnectedOutLayers()]
# 设置目标类别
classes = ['person', 'car', 'bus', 'truck']
# 对图像进行预处理
blob = cv2.dnn.blobFromImage(img, scalefactor=1/255, size=(416, 416), swapRB=True, crop=False)
# 将图像输入模型进行推理
model.setInput(blob)
outputs = model.forward(output_layers)
# 解析输出结果
conf_threshold = 0.5 # 置信度阈值
nms_threshold = 0.4 # 非极大值抑制阈值
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > conf_threshold:
center_x = int(detection[0] * img.shape[1])
center_y = int(detection[1] * img.shape[0])
w = int(detection[2] * img.shape[1])
h = int(detection[3] * img.shape[0])
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 对检测结果进行非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, conf_threshold, nms_threshold)
# 绘制检测结果
font = cv2.FONT_HERSHEY_PLAIN
for i in range(len(boxes)):
if i in indices:
x, y, w, h = boxes[i]
label = f'{classes[class_ids[i]]}: {confidences[i]:.2f}'
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(img, label, (x, y - 5), font, 1, (0, 255, 0), 2)
# 显示检测结果
cv2.imshow('object detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个程序使用了YOLOv3模型进行目标检测,并且可以检测人、车、公交车和卡车这四种类别。你需要将程序中的路径替换成你的图像和模型的路径。运行程序后,它将显示检测结果的图像。
阅读全文
相关推荐
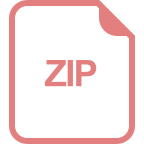
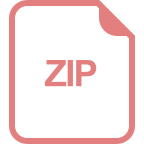
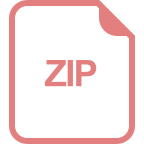
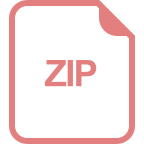
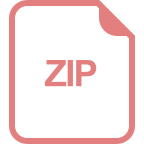
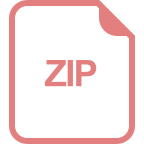
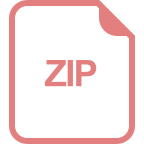
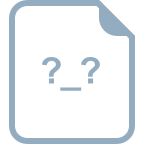
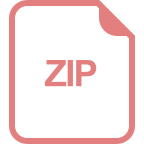
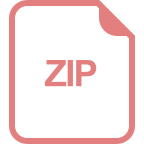
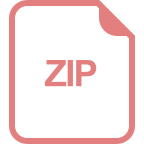
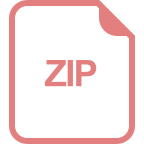
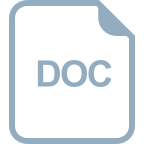
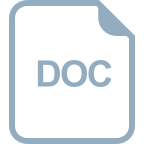
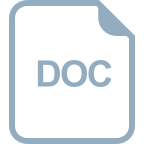
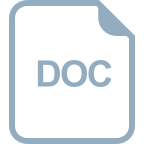