mybatis collection 示例
时间: 2023-10-29 09:07:16 浏览: 111
Sure, here's an example of using MyBatis collection in Java:
Suppose we have two classes, Blog and Post, where a Blog can have multiple Posts. We want to retrieve all the Blogs and their respective Posts using MyBatis.
First, we need to define the mappings for the two classes in the MyBatis XML configuration file:
```
<resultMap id="blogMap" type="Blog">
<id property="id" column="id" />
<result property="name" column="name" />
<collection property="posts" ofType="Post">
<id property="id" column="post_id" />
<result property="title" column="title" />
<result property="content" column="content" />
</collection>
</resultMap>
<resultMap id="postMap" type="Post">
<id property="id" column="id" />
<result property="title" column="title" />
<result property="content" column="content" />
</resultMap>
```
In the first resultMap, we define the mapping for the Blog class and its collection of Posts. In the collection element, we specify the property to which the collection should be mapped (in this case, "posts"), the type of the collection element (Post), and the mappings for the collection element.
In the second resultMap, we define the mapping for the Post class.
Next, we can define the SQL statement to retrieve the data:
```
<select id="getAllBlogs" resultMap="blogMap">
SELECT b.id, b.name, p.id AS post_id, p.title, p.content
FROM blog b
LEFT JOIN post p ON b.id = p.blog_id
ORDER BY b.id ASC
</select>
```
Finally, we can use the MyBatis mapper interface to call the SQL statement and retrieve the data:
```
public interface BlogMapper {
@Select("getAllBlogs")
@ResultMap("blogMap")
List<Blog> getAllBlogs();
}
```
With this setup, calling the getAllBlogs() method on the BlogMapper interface will return a List of Blogs, each with its collection of Posts populated.
阅读全文
相关推荐
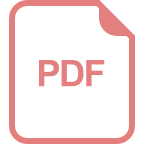

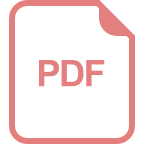
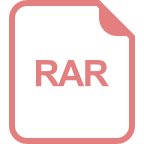
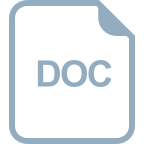
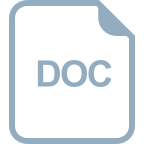









