js怎么合并两个List
时间: 2024-05-06 13:17:15 浏览: 165
可以使用 `concat()` 方法将两个数组合并成一个新数组,例如:
```javascript
const list1 = [1, 2, 3];
const list2 = [4, 5, 6];
const mergedList = list1.concat(list2);
console.log(mergedList); // Output: [1, 2, 3, 4, 5, 6]
```
另外,也可以使用扩展运算符 `...` 将两个数组合并成一个新数组,例如:
```javascript
const list1 = [1, 2, 3];
const list2 = [4, 5, 6];
const mergedList = [...list1, ...list2];
console.log(mergedList); // Output: [1, 2, 3, 4, 5, 6]
```
都可以实现合并两个数组的效果。
相关问题
js两个list合并
在JavaScript中合并两个数组(通常称为list)可以使用多种方法。以下是两种常用的方法:
1. 使用扩展运算符(Spread Operator):
扩展运算符可以将一个数组的所有元素复制到另一个新的数组中。通过这种方式,可以轻松地合并两个数组。
```javascript
let list1 = [1, 2, 3];
let list2 = [4, 5, 6];
let mergedList = [...list1, ...list2]; // 结果为 [1, 2, 3, 4, 5, 6]
```
2. 使用concat方法:
`concat`方法用于合并两个或多个数组。此方法不会改变现有的数组,而是返回一个新的数组,其中包含原始数组的元素。
```javascript
let list1 = [1, 2, 3];
let list2 = [4, 5, 6];
let mergedList = list1.concat(list2); // 结果为 [1, 2, 3, 4, 5, 6]
```
需要注意的是,使用`concat`方法合并数组时,如果数组中还包含其他数组,这些数组会被视为单独的元素合并到新数组中,而不是继续合并它们的内容。
javascript 两个list合并
在JavaScript中,将两个数组合并成一个新的数组通常有几种方法:
1. **`concat()`** 方法:这是一个原地不会改变原有数组的数组操作,它会创建并返回一个新数组,包含所有给定数组的所有元素。例如:
```javascript
let list1 = [1, 2, 3];
let list2 = ['a', 'b', 'c'];
let mergedList = list1.concat(list2); // mergedList will be [1, 2, 3, 'a', 'b', 'c']
```
2. **`push()` 和 `unshift()`** 方法:如果数组长度允许,也可以通过循环逐个添加元素到其中一个数组中,但这可能会改变原始数组的长度:
```javascript
let list1 = [1, 2, 3];
let list2 = ['a', 'b', 'c'];
for(let i = 0; i < list2.length; i++) {
list1.push(list2[i]);
}
```
3. **扩展运算符 (`...`) 或 `Array.from()`**: 这些方法也适用于简单的合并:
```javascript
let list1 = [1, 2, 3];
let list2 = ['a', 'b', 'c'];
let mergedList = [...list1, ...list2]; // same result as concat()
```
4. 使用 `Array.prototype.slice.call()` 或 `apply()` 方法,可以处理非数组类型的参数,如Node.js中的arguments对象:
```javascript
let list1 = [1, 2, 3];
let list2 = {0: 'a', 1: 'b', length: 2};
let mergedList = Array.prototype.slice.call(list1).concat(Array.prototype.slice.call(list2));
```
阅读全文
相关推荐
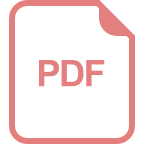













