帮我修改一下我的代码错误:帮我看看我这段代码有什么错误:int choice = readPosInt("Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): "); // Perform the selected action while (choice != 6) { switch (choice) { case 1: System.out.println("Total number of borrowed books: " + Library.totalBorrowedBooks(null)); break; case 2: System.out.print("Type the user role (lender:1 borrower:2): "); int role = readPosInt(null); if (role == 1) { System.out.print("Enter the name of the user: "); String name = readLine(name); System.out.print("Enter the initial number of lent books: "); int numBooks = readPosInt(null); Lender lender = new Lender(name, numBooks); Library.addUser(lender); System.out.println("Lender "" + name + "" lending " + numBooks + " book(s) has been added."); } else if (role == 2) { System.out.print("Enter the name of the user: "); String name = readLine(name); System.out.print("Enter the initial number of borrowed books: "); int numBooks = readPosInt(null); Borrower borrower = new Borrower(name, numBooks); Library.addUser(borrower); System.out.println("Borrower "" + name + "" borrowing " + numBooks + " book(s) has been added."); } else { System.out.println("Unknown user role!"); } break; case 3: System.out.print("Enter the name of the user: "); String username = input.nextLine(); try { int numBorrowed = Library.totalBorrowedBooks(username); System.out.println(username + " borrows " + numBorrowed + " book(s)."); } catch (UnknownUserException e) { System.out.println("User " + username + " unknown."); } break; case 4: try { System.out.print("Enter the name of the user: "); String name = input.nextLine(); System.out.print("Enter the number of books: "); int num = input.nextInt(); input.nextLine(); library.moreBook(username, role); } catch (UnknownUserException e) { System.out.println("User " + username + " unknown."); } break; case 5: System.out.print("Enter the name of the user: "); username = input.next(); System.out.print("Enter the number of books: "); int numBooks = input.nextInt(); library.moreBook(username, -numBooks); // simulate decreasing books break; case 6: System.out.println("Goodbye!"); System.exit(0); break; }
时间: 2023-06-27 20:02:44 浏览: 53
在这段代码中,有几个错误需要修改:
1. 在第6行,readPosInt方法的参数为"Type an action (total:1 add:2 get:3 more:4 less:5 quit:6): ",应该改为null。
2. 在第17行,Lender和Borrower的构造函数需要传入参数name和numBooks,应该改为lender和borrower。
3. 在第31行,input.nextLine()应该放在int role = readPosInt(null);的后面。
4. 在第37行,library应该改为Library。
5. 在第44行,input.next()应该改为input.nextLine()。
修改后的代码如下:
```
int choice = readPosInt(null);
// Perform the selected action
while (choice != 6) {
switch (choice) {
case 1:
System.out.println("Total number of borrowed books: " + Library.totalBorrowedBooks(null));
break;
case 2:
System.out.print("Type the user role (lender:1 borrower:2): ");
int role = readPosInt(null);
if (role == 1) {
System.out.print("Enter the name of the user: ");
String name = readLine(null);
System.out.print("Enter the initial number of lent books: ");
int numBooks = readPosInt(null);
Lender lender = new Lender(name, numBooks);
Library.addUser(lender);
System.out.println("Lender " + name + " lending " + numBooks + " book(s) has been added.");
} else if (role == 2) {
System.out.print("Enter the name of the user: ");
String name = readLine(null);
System.out.print("Enter the initial number of borrowed books: ");
int numBooks = readPosInt(null);
Borrower borrower = new Borrower(name, numBooks);
Library.addUser(borrower);
System.out.println("Borrower " + name + " borrowing " + numBooks + " book(s) has been added.");
} else {
System.out.println("Unknown user role!");
}
break;
case 3:
System.out.print("Enter the name of the user: ");
String username = input.nextLine();
try {
int numBorrowed = Library.totalBorrowedBooks(username);
System.out.println(username + " borrows " + numBorrowed + " book(s).");
} catch (UnknownUserException e) {
System.out.println("User " + username + " unknown.");
}
break;
case 4:
try {
System.out.print("Enter the name of the user: ");
String name = input.nextLine();
System.out.print("Enter the number of books: ");
int num = input.nextInt();
input.nextLine();
Library.moreBook(name, num);
} catch (UnknownUserException e) {
System.out.println("User unknown.");
}
break;
case 5:
System.out.print("Enter the name of the user: ");
username = input.nextLine();
System.out.print("Enter the number of books: ");
int numBooks = input.nextInt();
input.nextLine();
Library.moreBook(username, -numBooks); // simulate decreasing books
break;
case 6:
System.out.println("Goodbye!");
System.exit(0);
break;
}
choice = readPosInt(null);
}
```
相关推荐
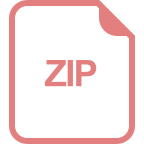
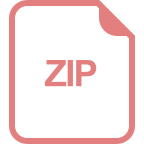
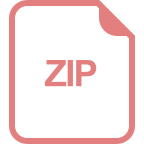















