Pthreads梯形积分法
时间: 2023-09-14 17:05:16 浏览: 56
Pthreads梯形积分法是一种使用Pthreads多线程技术实现的数值积分方法。该方法将积分区间划分为若干个子区间,在每个子区间上使用梯形公式求解积分值,并将各个子区间的积分值相加得到最终的积分值。
在Pthreads梯形积分法中,每个线程负责计算一个子区间的积分值。线程之间可以通过共享变量来协调计算任务,比如共享积分结果、共享当前计算的区间等。
该方法的优点是可以充分利用多核CPU的计算能力,提高计算效率。同时,Pthreads多线程技术也是一种常用的并行编程技术,在其他计算密集型任务中也有广泛的应用。
相关问题
用Pthreads实现梯形积分法
梯形积分法是一种数值积分方法,可以用来近似计算定积分。在使用Pthreads实现梯形积分法时,可以将计算任务分成多个子任务,每个子任务使用梯形积分法来计算一部分定积分,然后将所有子任务的计算结果汇总得到最终的积分值。
下面是一个使用Pthreads实现梯形积分法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <math.h>
#define NUM_THREADS 4 // 线程数量
#define N 10000000 // 划分区间数
#define a 0.0 // 积分下限
#define b 1.0 // 积分上限
double f(double x) {
return sin(x); // 被积函数
}
double trap(double left, double right, double height) {
return (left + right) * height / 2.0; // 梯形面积公式
}
void *worker(void *arg) {
int tid = *(int*)arg;
double h = (b - a) / N; // 子区间宽度
int start = tid * N / NUM_THREADS; // 子区间起始索引
int end = (tid + 1) * N / NUM_THREADS; // 子区间结束索引
double sum = 0.0;
for (int i = start; i < end; i++) {
double left = a + i * h;
double right = a + (i + 1) * h;
double height = (f(left) + f(right)) / 2.0;
sum += trap(f(left), f(right), h);
}
double *result = malloc(sizeof(double));
*result = sum;
return (void*)result;
}
int main() {
pthread_t threads[NUM_THREADS];
int tids[NUM_THREADS];
double sum = 0.0;
for (int i = 0; i < NUM_THREADS; i++) {
tids[i] = i;
pthread_create(&threads[i], NULL, worker, (void*)&tids[i]);
}
for (int i = 0; i < NUM_THREADS; i++) {
double *result;
pthread_join(threads[i], (void**)&result);
sum += *result;
free(result);
}
printf("Integral value: %f\n", sum);
return 0;
}
```
在这个示例中,我们定义了一个`worker`函数来执行每个子任务的计算。该函数需要接受一个整数参数,表示线程的ID。在该函数中,我们首先计算出子区间的起始索引和结束索引,然后通过梯形积分法来计算该子区间的积分值。最后,将计算结果存储在堆上分配的内存中,并将其作为返回值返回。
在`main`函数中,我们首先创建了多个线程,并分别为每个线程传递一个唯一的线程ID。然后,我们使用`pthread_join`函数来等待每个线程的计算结果,并将这些结果相加得到最终的积分值。最后,我们输出积分值并退出程序。
需要注意的是,在使用Pthreads实现梯形积分法时,需要将任务平均分配给每个线程,并确保每个线程都处理不同的区间。此外,由于每个线程都会分配堆上的内存,因此在使用完毕后需要及时释放这些内存,以避免内存泄漏问题。
用pthreads实现梯形积分法
梯形积分法是一种数值积分方法,可以用于数值求解函数的积分。下面是用pthreads实现梯形积分法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NTHREADS 4
double a = 0.0, b = 1.0;
int n = 10000000;
double h;
double result = 0.0;
pthread_mutex_t mutex;
void* trapezoid_rule(void* rank) {
long my_rank = (long) rank;
int local_n = n / NTHREADS;
double local_a = a + my_rank * local_n * h;
double local_b = local_a + local_n * h;
double local_result = 0.0;
double x_i;
for (int i = 0; i < local_n; i++) {
x_i = local_a + i * h;
local_result += (f(x_i) + f(x_i + h)) * h / 2.0;
}
pthread_mutex_lock(&mutex);
result += local_result;
pthread_mutex_unlock(&mutex);
return NULL;
}
double f(double x) {
return x * x;
}
int main(void) {
pthread_t threads[NTHREADS];
h = (b - a) / n;
pthread_mutex_init(&mutex, NULL);
for (long rank = 0; rank < NTHREADS; rank++) {
pthread_create(&threads[rank], NULL, trapezoid_rule, (void*) rank);
}
for (long rank = 0; rank < NTHREADS; rank++) {
pthread_join(threads[rank], NULL);
}
pthread_mutex_destroy(&mutex);
printf("Result: %lf\n", result);
return 0;
}
```
在这个示例代码中,我们使用了四个线程计算积分。每个线程计算一个局部积分,最后将局部积分相加得到整个积分。为了保证线程安全,我们使用了互斥锁来控制对共享变量`result`的访问。
相关推荐
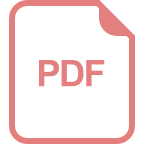




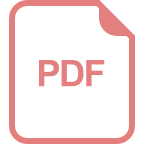
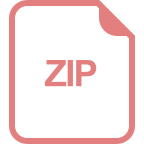