有10个学生,每个学生的数据包括学号、姓名、3门课程的成绩。从键盘输入10个学生的数据,要求输出3门课程的总平均成绩,以及最高分的学生的学号、姓名、3门课程成绩、平均分数。
时间: 2023-12-15 16:24:57 浏览: 37
好的,我可以帮你写一个 Python 程序来实现这个功能。以下是代码:
```python
# 定义学生类
class Student:
def __init__(self, id, name, score1, score2, score3):
self.id = id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
# 计算总成绩
def total_score(self):
return self.score1 + self.score2 + self.score3
# 计算平均成绩
def avg_score(self):
return self.total_score() / 3
# 定义学生列表
students = []
# 循环输入学生数据
for i in range(10):
id = input("请输入第{}个学生的学号:".format(i+1))
name = input("请输入第{}个学生的姓名:".format(i+1))
score1 = float(input("请输入第{}个学生的第1门课程成绩:".format(i+1)))
score2 = float(input("请输入第{}个学生的第2门课程成绩:".format(i+1)))
score3 = float(input("请输入第{}个学生的第3门课程成绩:".format(i+1)))
# 创建学生对象并添加到列表中
student = Student(id, name, score1, score2, score3)
students.append(student)
# 计算3门课程的总平均成绩
total_score = 0
for student in students:
total_score += student.total_score()
avg_score = total_score / (10 * 3)
# 找出最高分的学生
max_score_student = max(students, key=lambda x: x.total_score())
# 输出结果
print("3门课程的总平均成绩为:{:.2f}".format(avg_score))
print("最高分的学生信息如下:")
print("学号:", max_score_student.id)
print("姓名:", max_score_student.name)
print("第1门课程成绩:{:.2f}".format(max_score_student.score1))
print("第2门课程成绩:{:.2f}".format(max_score_student.score2))
print("第3门课程成绩:{:.2f}".format(max_score_student.score3))
print("平均分数:{:.2f}".format(max_score_student.avg_score()))
```
运行程序后,可以按照提示输入10个学生的数据,程序会计算出3门课程的总平均成绩,以及最高分的学生的学号、姓名、3门课程成绩、平均分数,并输出结果。
相关推荐
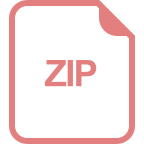
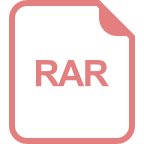














