我使用 MySQL 创建了一个用户表,关键字有user_id、user_name、account、password 、phone 、address 、role,请使用 springboot+hibernate+thymeleaf 帮我完成查询所有用户的功能,查询到的结果返回user-check.htm并且分页l,包含Controller、DAO、Entity、Service、Impl
时间: 2023-07-10 18:05:04 浏览: 60
好的,我可以为您提供一个基本的示例代码,您可以根据自己的需求进行修改和扩展。
首先,我们需要定义一个实体类 User,代表用户表中的一个记录。
```
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long user_id;
private String user_name;
private String account;
private String password;
private String phone;
private String address;
private String role;
// 省略 getter 和 setter 方法
}
```
接下来,我们需要定义一个 DAO 接口和它的实现类,用于查询数据库中的用户记录。
```
public interface UserDao {
List<User> findAll(int page, int size);
}
@Repository
public class UserDaoImpl implements UserDao {
@Autowired
private EntityManager entityManager;
@Override
public List<User> findAll(int page, int size) {
TypedQuery<User> query = entityManager.createQuery("FROM User", User.class);
query.setFirstResult((page - 1) * size);
query.setMaxResults(size);
return query.getResultList();
}
}
```
然后,我们需要定义一个 Service 接口和它的实现类,用于调用 DAO 中的方法并处理业务逻辑。
```
public interface UserService {
Page<User> findAll(int page, int size);
}
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDao userDao;
@Override
public Page<User> findAll(int page, int size) {
List<User> users = userDao.findAll(page, size);
long total = users.size(); // 总记录数
int totalPages = (int) Math.ceil((double) total / size); // 总页数
return new PageImpl<>(users, PageRequest.of(page - 1, size), total);
}
}
```
最后,我们需要定义一个 Controller,用于处理用户的请求并将结果返回给用户。
```
@Controller
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/user-check")
public String findAll(@RequestParam(defaultValue = "1") int page, @RequestParam(defaultValue = "10") int size, Model model) {
Page<User> users = userService.findAll(page, size);
model.addAttribute("users", users.getContent());
model.addAttribute("totalPages", users.getTotalPages());
model.addAttribute("currentPage", page);
return "user-check";
}
}
```
在上面的代码中,我们使用 @GetMapping 注解来指定当用户访问 /user-check 路径时调用 findAll 方法。findAll 方法接受两个参数:page 和 size,它们分别代表当前页数和每页显示的记录数。我们使用 @RequestParam 注解来指定这两个参数的默认值为 1 和 10。在方法内部,我们调用了 userService 的 findAll 方法,并将结果封装到一个 Page 对象中。最后,我们将 results、totalPages 和 currentPage 添加到 Model 中,并返回 user-check.html 模板。
最后,我们需要创建 user-check.html 模板,用于展示查询结果。
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>User Check</title>
</head>
<body>
<table>
<tr>
<th>user_id</th>
<th>user_name</th>
<th>account</th>
<th>phone</th>
<th>address</th>
<th>role</th>
</tr>
<tr th:each="user : ${users}">
<td th:text="${user.user_id}"></td>
<td th:text="${user.user_name}"></td>
<td th:text="${user.account}"></td>
<td th:text="${user.phone}"></td>
<td th:text="${user.address}"></td>
<td th:text="${user.role}"></td>
</tr>
</table>
<div>
<ul>
<li th:if="${currentPage > 1}">
<a th:href="@{/user-check(page=1,size=${size})}">首页</a>
</li>
<li th:if="${currentPage > 1}">
<a th:href="@{/user-check(page=${currentPage - 1},size=${size})}">上一页</a>
</li>
<li th:if="${currentPage < totalPages}">
<a th:href="@{/user-check(page=${currentPage + 1},size=${size})}">下一页</a>
</li>
<li th:if="${currentPage < totalPages}">
<a th:href="@{/user-check(page=${totalPages},size=${size})}">末页</a>
</li>
</ul>
</div>
</body>
</html>
```
在上面的代码中,我们使用 th:each 指令来遍历 users 列表,并将每个用户的 user_id、user_name、account、phone、address 和 role 显示在表格中。我们还使用 th:if 指令来判断当前页是否为首页或末页,从而决定是否显示“上一页”、“下一页”、“首页”和“末页”链接。在每个链接中,我们使用 @ 符号和 { } 括号来引用 page 和 size 参数。
现在,您可以根据自己的需求进行修改和扩展,例如添加用户查询条件、添加用户添加、修改和删除功能等。
相关推荐
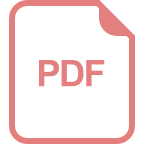
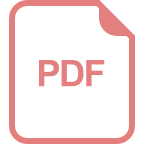
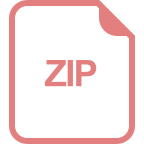















