stm32c8t6八位数码管显示
时间: 2023-10-09 08:06:46 浏览: 111
要控制八位数码管,你需要了解以下几个方面:
1. 数码管的工作原理:数码管是由多个LED组成,每个LED代表不同的数字或字符。通过对不同的LED进行亮灭控制,可以显示不同的数字或字符。
2. 数码管的接口:通常,数码管的接口包括8个控制引脚和1个共阴或共阳引脚。其中,8个控制引脚分别对应8个LED,用于控制它们的亮灭;共阴或共阳引脚则用于控制所有LED的共性端。
3. 控制方法:控制数码管的方法有很多种,常见的方式包括直接控制、定时器控制和中断控制等。在这里,我们以直接控制为例。
下面是一个简单的用STM32C8T6控制8位数码管的代码示例,假设使用的是共阴数码管:
```c
#include "stm32f10x.h"
#define GPIO_LED_PORT GPIOA
#define GPIO_LED_PIN_0 GPIO_Pin_0
#define GPIO_LED_PIN_1 GPIO_Pin_1
#define GPIO_LED_PIN_2 GPIO_Pin_2
#define GPIO_LED_PIN_3 GPIO_Pin_3
#define GPIO_LED_PIN_4 GPIO_Pin_4
#define GPIO_LED_PIN_5 GPIO_Pin_5
#define GPIO_LED_PIN_6 GPIO_Pin_6
#define GPIO_LED_PIN_7 GPIO_Pin_7
void delay(uint32_t count)
{
for(uint32_t i=0;i<count;i++);
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6 | GPIO_LED_PIN_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIO_LED_PORT, &GPIO_InitStructure);
while(1)
{
GPIO_SetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6 | GPIO_LED_PIN_7);
// 显示数字0
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_5);
delay(1000);
// 显示数字1
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_1 | GPIO_LED_PIN_2);
delay(1000);
// 显示数字2
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字3
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字4
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字5
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字6
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字7
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2);
delay(1000);
// 显示数字8
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_4 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6);
delay(1000);
// 显示数字9
GPIO_ResetBits(GPIO_LED_PORT, GPIO_LED_PIN_0 | GPIO_LED_PIN_1 | GPIO_LED_PIN_2 | GPIO_LED_PIN_3 | GPIO_LED_PIN_5 | GPIO_LED_PIN_6);
delay(1000);
}
}
```
这个示例代码中,GPIO_LED_PORT对应的是数码管的8个控制引脚,GPIO_LED_PIN_0至GPIO_LED_PIN_7分别对应8个LED。在while循环中,通过依次控制每个LED的亮灭,来实现8位数码管的显示。
阅读全文
相关推荐
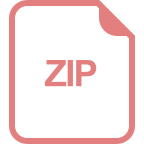
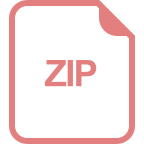
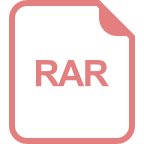



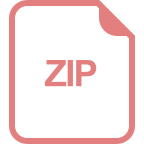
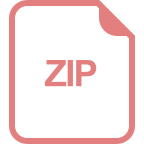
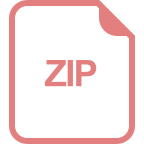
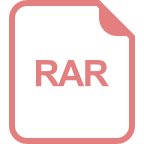
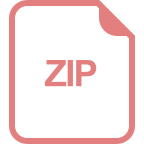
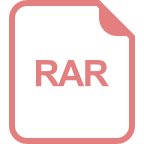
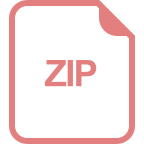
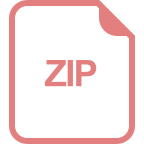
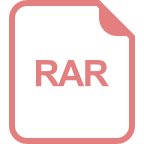
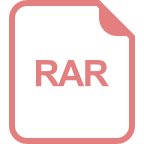
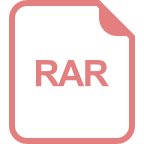