生成一个调试图像并应用yolo v4进入目标测试的python代码
时间: 2024-05-02 20:22:03 浏览: 92
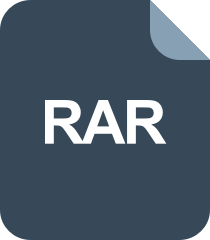
基于python与yolo v4算法模型的目标跟踪算法设计与实现
以下是一个生成调试图像并应用yolo v4进行目标检测的Python代码示例:
```python
import cv2
import numpy as np
# 加载yolo v4模型和类别名称
net = cv2.dnn.readNet("yolov4.weights", "yolov4.cfg")
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 生成调试图像
img = np.zeros((512, 512, 3), np.uint8)
img[:, 256:, :] = (0, 255, 0)
img[:256, :, :] = (0, 0, 255)
# 运行yolo v4目标检测
blob = cv2.dnn.blobFromImage(img, 1 / 255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
# 解析检测结果并绘制边界框
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * 512)
center_y = int(detection[1] * 512)
w = int(detection[2] * 512)
h = int(detection[3] * 512)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2)
cv2.putText(img, classes[class_id], (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 0, 0), 2)
# 显示结果图像
cv2.imshow("Debug Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
此代码将生成一个大小为512x512的调试图像,并在图像的右半部分绘制绿色,上半部分绘制红色。然后,它将yolo v4模型应用于该图像,并在检测到的任何目标周围绘制边界框。最后,它将显示结果图像。您需要将yolov4.weights,yolov4.cfg和coco.names文件放在同一目录下,以便代码可以加载它们。
阅读全文
相关推荐
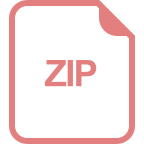
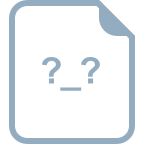
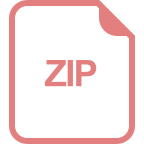
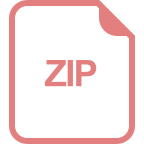
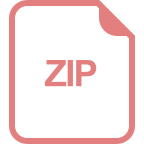
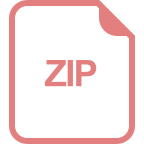
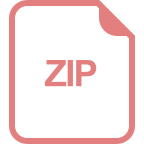
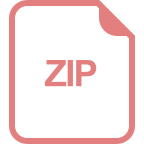
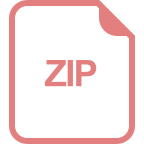
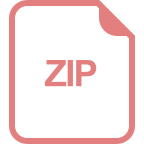
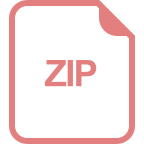
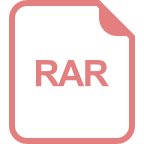
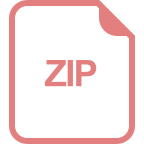
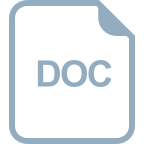