c++连接mysql数据库
时间: 2023-09-13 16:08:10 浏览: 132
要在 C++ 中连接 MySQL 数据库,需要使用 MySQL Connector/C++,这是一个官方提供的 C++ 连接器。
以下是连接 MySQL 数据库的步骤:
1. 下载并安装 MySQL Connector/C++。
2. 包含头文件和库文件。
```c++
#include <mysqlx/xdevapi.h>
```
3. 建立连接。
```c++
mysqlx::Session session("localhost", 33060, "root", "password");
```
其中,`localhost` 为主机名,`33060` 为端口号,`root` 为用户名,`password` 为密码。
4. 执行 SQL 语句。
```c++
mysqlx::Schema db = session.getSchema("mydatabase");
mysqlx::Table mytable = db.getTable("mytable");
mysqlx::RowResult result = mytable.select("*").execute();
```
其中,`mydatabase` 和 `mytable` 分别为数据库和表的名称。
5. 处理查询结果。
```c++
while (auto row = result.fetchOne()) {
std::cout << row[0] << ", " << row[1] << ", " << row[2] << std::endl;
}
```
完整示例代码如下:
```c++
#include <mysqlx/xdevapi.h>
#include <iostream>
int main() {
try {
mysqlx::Session session("localhost", 33060, "root", "password");
mysqlx::Schema db = session.getSchema("mydatabase");
mysqlx::Table mytable = db.getTable("mytable");
mysqlx::RowResult result = mytable.select("*").execute();
while (auto row = result.fetchOne()) {
std::cout << row[0] << ", " << row[1] << ", " << row[2] << std::endl;
}
} catch(const mysqlx::Error &err) {
std::cout << "Error: " << err << std::endl;
}
return 0;
}
```
注意,这只是一个基本的示例,实际应用中可能需要更复杂的 SQL 查询语句和结果处理方式。
阅读全文
相关推荐


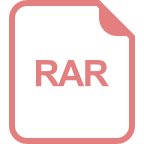


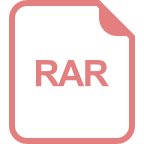




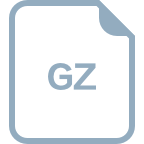