用单链表写房屋销售管理信息系统的设计与实现 (1)房屋信息包括:房屋号(char num[10])、售房者姓名(char name1[10])、中介职工姓名(char name2[10])、房屋销售价格(float money) (2)除了总的模块要求外,还需计算超过200万的房屋总数,并输出这些房屋的详细信息。
时间: 2024-03-21 15:41:45 浏览: 19
好的,这是一个非常好的编程问题。我们可以按照以下步骤进行实现:
1. 定义单链表节点结构体,包括房屋号、售房者姓名、中介职工姓名、房屋销售价格和指向下一个节点的指针。
```C++
struct HouseNode {
char num[10]; // 房屋号
char name1[10]; // 售房者姓名
char name2[10]; // 中介职工姓名
float money; // 房屋销售价格
HouseNode* next; // 指向下一个节点的指针
};
```
2. 定义单链表类,包括头节点指针以及插入节点、遍历链表、计算超过200万的房屋总数并输出详细信息的方法。
```C++
class HouseList {
public:
HouseList(); // 构造函数,初始化头节点指针为 NULL
void InsertNode(char num[10], char name1[10], char name2[10], float money); // 插入节点方法
void TraverseList(); // 遍历链表方法
void CountAndPrint(); // 计算超过200万的房屋总数并输出详细信息方法
private:
HouseNode* head; // 头节点指针
};
```
3. 在类的实现中,定义构造函数,将头节点指针初始化为 NULL。
```C++
HouseList::HouseList() {
head = NULL;
}
```
4. 定义插入节点方法,创建新节点并将其插入链表中。
```C++
void HouseList::InsertNode(char num[10], char name1[10], char name2[10], float money) {
HouseNode* newNode = new HouseNode;
strcpy(newNode->num, num);
strcpy(newNode->name1, name1);
strcpy(newNode->name2, name2);
newNode->money = money;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
HouseNode* currentNode = head;
while (currentNode->next != NULL) {
currentNode = currentNode->next;
}
currentNode->next = newNode;
}
}
```
5. 定义遍历链表方法,用于输出链表中所有节点的信息。
```C++
void HouseList::TraverseList() {
HouseNode* currentNode = head;
while (currentNode != NULL) {
cout << "房屋号:" << currentNode->num << endl;
cout << "售房者姓名:" << currentNode->name1 << endl;
cout << "中介职工姓名:" << currentNode->name2 << endl;
cout << "房屋销售价格:" << currentNode->money << endl << endl;
currentNode = currentNode->next;
}
}
```
6. 定义计算超过200万的房屋总数并输出详细信息方法,遍历链表并计算超过200万的房屋总数,同时输出这些房屋的详细信息。
```C++
void HouseList::CountAndPrint() {
int count = 0; // 超过200万的房屋总数
HouseNode* currentNode = head;
while (currentNode != NULL) {
if (currentNode->money > 2000000) {
count++;
cout << "房屋号:" << currentNode->num << endl;
cout << "售房者姓名:" << currentNode->name1 << endl;
cout << "中介职工姓名:" << currentNode->name2 << endl;
cout << "房屋销售价格:" << currentNode->money << endl << endl;
}
currentNode = currentNode->next;
}
cout << "超过200万的房屋总数为:" << count << endl;
}
```
7. 最后,我们可以在主函数中创建一个 HouseList 对象,并测试插入节点、遍历链表、计算超过200万的房屋总数并输出详细信息的功能。
```C++
int main() {
HouseList houseList;
houseList.InsertNode("001", "张三", "李四", 1500000);
houseList.InsertNode("002", "王五", "赵六", 2200000);
houseList.InsertNode("003", "钱七", "孙八", 1800000);
houseList.InsertNode("004", "周九", "吴十", 2500000);
houseList.TraverseList();
houseList.CountAndPrint();
return 0;
}
```
这样,我们就完成了用单链表写房屋销售管理信息系统的设计与实现。
相关推荐
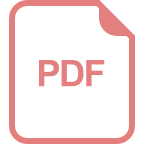
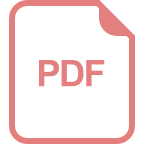
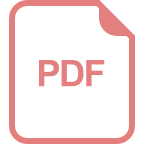
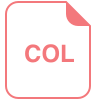
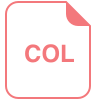
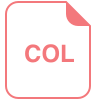
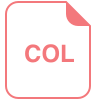
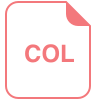










