用Delphi实现一个完成 多段线去除共线边 的算法。有以下要点 图形都是二维平面上的 多段线是用顶点列表来表示的,可以自交,可以不封闭(如果是封闭的,首尾点一定是重合的,这种重合点不必去除) 共线边分为3种,如果相邻两条边表示为AB、BC,则有 ① AB重合 ② AB、BC 同向 ③ AB、BC 反向 去除共线边的方式为删除B点即可 可能并不需要同时处理所有情况,函数会有参数控制是否去除同向边和反向边 只剩<=2个顶点时不必再简化了 浮点数计算都会有误差,因此几乎不存在完全同向反向的边,我们提供一个容差,通过判断相邻两边的叉积与容差的关系来近似判断是否同向反向(也兼容了重合点的判断)。 当然这种方式也有弊端,很短的边夹角很大也可能被简化,但我们暂时接受这个缺点。 可能注释中不会写,但算法应当满足基本的认知,例如: 算法不应改变多边形的封闭性 简化应当彻底,即对第一次算法的结果再调用一次,不能继续简化 …… 这是底层算法,需要保证: Delphi2010 、Delphi11 都可以正确运行 32位、64位都可以正确运行
时间: 2024-03-09 19:51:01 浏览: 50
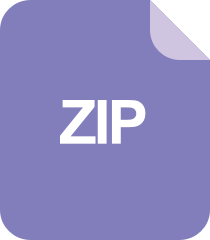
delphi 多线程
以下是一个用 Delphi 实现多段线去除共线边的算法的示例代码:
```delphi
type
TPoint = record
X: Double;
Y: Double;
end;
TPolyline = array of TPoint;
function SimplifyPolyline(const Polyline: TPolyline; Tolerance: Double; RemoveSameDirection, RemoveOppositeDirection: Boolean): TPolyline;
var
i, j, k: Integer;
dx1, dy1, dx2, dy2, cross: Double;
Points: TPolyline;
begin
SetLength(Points, Length(Polyline));
for i := 0 to High(Polyline) do
Points[i] := Polyline[i];
i := 0;
while (Length(Points) > 2) and (i < Length(Points) - 1) do
begin
dx1 := Points[i + 1].X - Points[i].X;
dy1 := Points[i + 1].Y - Points[i].Y;
dx2 := Points[i + 2].X - Points[i + 1].X;
dy2 := Points[i + 2].Y - Points[i + 1].Y;
cross := dx1 * dy2 - dy1 * dx2;
if (Abs(cross) < Tolerance) and (
(RemoveSameDirection and ((dx1 * dx2 > 0) or (dy1 * dy2 > 0))) or
(RemoveOppositeDirection and ((dx1 * dx2 < 0) or (dy1 * dy2 < 0)))) then
begin
for j := i + 1 to High(Points) - 1 do
Points[j] := Points[j + 1];
SetLength(Points, Length(Points) - 1);
if i > 0 then
Dec(i);
end
else
Inc(i);
end;
Result := Points;
end;
```
这个算法的实现比较简单,它遍历多段线的所有节点,并对相邻的三个节点计算它们的连线的叉积。如果叉积小于一个给定的容差,并且相邻两条边符合要求(同向或反向),则删除中间的节点。如果多段线的长度小于等于 2,或者已经无法再删除节点,算法就结束了。
你可以根据自己的需要调整容差和删除同向或反向边的设置,以达到最佳的简化效果。
阅读全文
相关推荐
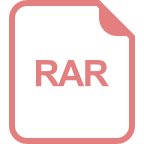
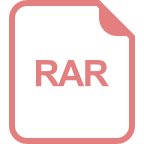
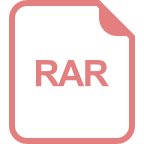
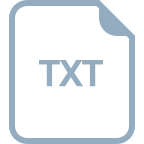
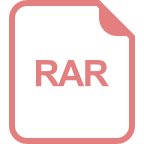
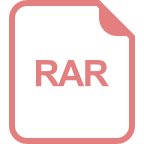
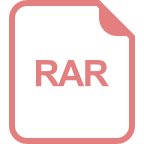
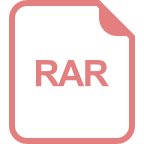
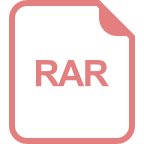
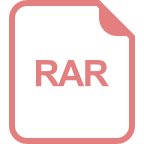
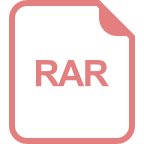
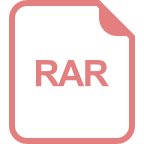