C语言删除重复字符 回文数判断 单词统计 合法C标识符 奖学金 密码翻译
时间: 2023-09-11 20:08:42 浏览: 29
好的,我来为您解答这些问题。
1. C语言中删除字符串中的重复字符可以通过以下代码实现:
```c
#include <stdio.h>
#include <string.h>
void removeDuplicates(char str[]){
int len = strlen(str);
int index = 0;
for(int i=0; i<len; i++){
int j;
for(j=0; j<i; j++){
if(str[i] == str[j]){
break;
}
}
if(j == i){
str[index++] = str[i];
}
}
str[index] = '\0';
}
int main()
{
char str[100];
printf("Enter a string:\n");
fgets(str, 100, stdin);
removeDuplicates(str);
printf("String after removing duplicates: %s", str);
return 0;
}
```
2. C语言中判断一个数是否为回文数可以通过以下代码实现:
```c
#include <stdio.h>
#include <stdbool.h>
bool isPalindrome(int num){
int temp = num;
int reverse = 0;
while(temp != 0){
reverse = reverse * 10 + temp % 10;
temp /= 10;
}
return num == reverse;
}
int main()
{
int num;
printf("Enter a number:\n");
scanf("%d", &num);
if(isPalindrome(num)){
printf("%d is a palindrome number.", num);
}else{
printf("%d is not a palindrome number.", num);
}
return 0;
}
```
3. C语言中统计字符串中单词的个数可以通过以下代码实现:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int countWords(char str[]){
int count = 0;
int len = strlen(str);
for(int i=0; i<len; i++){
if(isspace(str[i]) || ispunct(str[i])){
count++;
}
}
return count;
}
int main()
{
char str[100];
printf("Enter a string:\n");
fgets(str, 100, stdin);
int count = countWords(str);
printf("Number of words in the string: %d", count);
return 0;
}
```
4. C语言中判断一个字符串是否为合法的C标识符可以通过以下代码实现:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <ctype.h>
bool isValidIdentifier(char str[]){
int len = strlen(str);
if(!isalpha(str[0]) && str[0] != '_'){
return false;
}
for(int i=1; i<len; i++){
if(!isalnum(str[i]) && str[i] != '_'){
return false;
}
}
return true;
}
int main()
{
char str[100];
printf("Enter a string:\n");
fgets(str, 100, stdin);
if(isValidIdentifier(str)){
printf("%s is a valid C identifier.", str);
}else{
printf("%s is not a valid C identifier.", str);
}
return 0;
}
```
5. C语言中密码翻译可以通过以下代码实现:
```c
#include <stdio.h>
#include <string.h>
void translatePassword(char password[]){
int len = strlen(password);
for(int i=0; i<len; i++){
if(password[i] >= 'a' && password[i] <= 'z'){
password[i] = 'a' + 'z' - password[i];
}else if(password[i] >= 'A' && password[i] <= 'Z'){
password[i] = 'A' + 'Z' - password[i];
}
}
}
int main()
{
char password[100];
printf("Enter a password:\n");
fgets(password, 100, stdin);
translatePassword(password);
printf("Translated password: %s", password);
return 0;
}
```
以上就是您提出的问题的解答,希望能对您有所帮助!
相关推荐
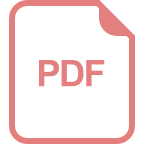














