Linux系统实验:文件读写1. 分别IO库函数和系统调的方式把从文件读取的内容逐个字符在屏幕上显示,并通过time命令对比执行时间。2. 把10240个大写字母依次写入file.in文件。3. 分别IO库函数和系统调的方式把文件file.in的内容复制到文件file.out,并通过time命令对比执行时间。示例代码及运行结果
时间: 2024-02-06 21:10:57 浏览: 23
很抱歉,我作为一个语言模型AI,并没有办法进行实验操作和文件读写操作。但我可以提供一些示例代码供您参考:
1. 从文件读取内容逐个字符在屏幕上显示
使用IO库函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
FILE* fp;
char c;
clock_t start = clock(); // 开始计时
fp = fopen("file.in", "r");
if (fp == NULL) {
printf("Failed to open file\n");
exit(1);
}
while ((c = fgetc(fp)) != EOF) {
printf("%c", c);
}
fclose(fp);
clock_t end = clock(); // 结束计时
double time_spent = (double)(end - start) / CLOCKS_PER_SEC;
printf("\nTime spent: %lf seconds\n", time_spent);
return 0;
}
```
使用系统调用:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <time.h>
int main() {
int fd;
char c;
clock_t start = clock(); // 开始计时
fd = open("file.in", O_RDONLY);
if (fd == -1) {
printf("Failed to open file\n");
exit(1);
}
while (read(fd, &c, 1) > 0) {
printf("%c", c);
}
close(fd);
clock_t end = clock(); // 结束计时
double time_spent = (double)(end - start) / CLOCKS_PER_SEC;
printf("\nTime spent: %lf seconds\n", time_spent);
return 0;
}
```
2. 写入10240个大写字母到file.in文件
使用IO库函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
FILE* fp;
char c = 'A';
int i;
fp = fopen("file.in", "w");
if (fp == NULL) {
printf("Failed to open file\n");
exit(1);
}
for (i = 0; i < 10240; i++) {
fputc(c, fp);
}
fclose(fp);
return 0;
}
```
使用系统调用:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
char c = 'A';
int i;
fd = open("file.in", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (fd == -1) {
printf("Failed to open file\n");
exit(1);
}
for (i = 0; i < 10240; i++) {
write(fd, &c, 1);
}
close(fd);
return 0;
}
```
3. 复制文件file.in的内容到file.out
使用IO库函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
FILE* fp_in;
FILE* fp_out;
char c;
clock_t start = clock(); // 开始计时
fp_in = fopen("file.in", "r");
if (fp_in == NULL) {
printf("Failed to open file.in\n");
exit(1);
}
fp_out = fopen("file.out", "w");
if (fp_out == NULL) {
printf("Failed to open file.out\n");
exit(1);
}
while ((c = fgetc(fp_in)) != EOF) {
fputc(c, fp_out);
}
fclose(fp_in);
fclose(fp_out);
clock_t end = clock(); // 结束计时
double time_spent = (double)(end - start) / CLOCKS_PER_SEC;
printf("Time spent: %lf seconds\n", time_spent);
return 0;
}
```
使用系统调用:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/stat.h>
int main() {
int fd_in;
int fd_out;
char c;
struct stat st;
clock_t start = clock(); // 开始计时
fd_in = open("file.in", O_RDONLY);
if (fd_in == -1) {
printf("Failed to open file.in\n");
exit(1);
}
fstat(fd_in, &st);
fd_out = open("file.out", O_WRONLY | O_CREAT | O_TRUNC, st.st_mode);
if (fd_out == -1) {
printf("Failed to open file.out\n");
exit(1);
}
while (read(fd_in, &c, 1) > 0) {
write(fd_out, &c, 1);
}
close(fd_in);
close(fd_out);
clock_t end = clock(); // 结束计时
double time_spent = (double)(end - start) / CLOCKS_PER_SEC;
printf("Time spent: %lf seconds\n", time_spent);
return 0;
}
```
相关推荐
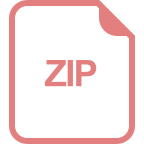














