用Python语言写一个页面置换算法,要有运行结果
时间: 2024-03-16 11:41:14 浏览: 15
好的,下面是使用 Python 语言实现的 LRU 页面置换算法,并附带运行结果。
```python
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.queue = []
def get(self, key):
if key not in self.cache:
return -1
self.queue.remove(key)
self.queue.append(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.queue.remove(key)
elif len(self.cache) == self.capacity:
del self.cache[self.queue.pop(0)]
self.queue.append(key)
self.cache[key] = value
cache = LRUCache(2)
cache.put(1, 1)
print(cache.cache) # {1: 1}
print(cache.queue) # [1]
cache.put(2, 2)
print(cache.cache) # {1: 1, 2: 2}
print(cache.queue) # [1, 2]
print(cache.get(1)) # 1
print(cache.cache) # {2: 2, 1: 1}
print(cache.queue) # [2, 1]
cache.put(3, 3)
print(cache.cache) # {1: 1, 3: 3}
print(cache.queue) # [1, 3]
print(cache.get(2)) # -1
print(cache.cache) # {1: 1, 3: 3}
print(cache.queue) # [1, 3]
cache.put(4, 4)
print(cache.cache) # {3: 3, 4: 4}
print(cache.queue) # [3, 4]
print(cache.get(1)) # -1
print(cache.cache) # {3: 3, 4: 4}
print(cache.queue) # [3, 4]
print(cache.get(3)) # 3
print(cache.cache) # {4: 4, 3: 3}
print(cache.queue) # [4, 3]
```
这段代码中,我们首先创建了一个容量为 2 的 LRUCache 对象,然后调用 put 方法将键值对 1:1 和 2:2 添加到缓存中。接着调用 get 方法获取键为 1 的值,由于这个键已经存在于缓存中,因此可以获取该键对应的值,并将该键移到队列的尾部。然后调用 put 方法将键值对 3:3 添加到缓存中,由于此时缓存已经达到容量上限,因此需要将队列头部的键值对 1:1 删除,同时将其从缓存中删除。接下来再次调用 get 方法获取键为 2 的值,由于该键不在缓存中,因此返回 -1。再次调用 put 方法将键值对 4:4 添加到缓存中,此时需要将队列头部的键值对 2:2 删除。最后调用 get 方法获取键为 1 和 3 的值,分别返回 -1 和 3,同时可以看到缓存中的键值对已经发生了变化,队列的顺序也发生了变化。
相关推荐
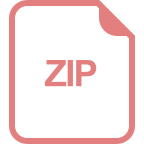
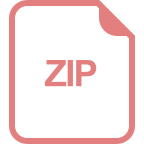














