vue使用ffmpeg视频压缩
时间: 2023-06-29 19:09:39 浏览: 191
Vue.js 是一个 JavaScript 的前端框架,它并不直接支持视频压缩功能。但是,我们可以使用 JavaScript 中的 FFmpeg 库,通过 Vue.js 来实现视频压缩的功能。
下面是使用 FFmpeg 库进行视频压缩的大致流程:
1. 将用户上传的视频文件读入到 JavaScript 中。
2. 在 JavaScript 中使用 FFmpeg 库来进行视频压缩。
3. 将压缩后的视频文件保存到服务器或者本地存储中。
下面是一个基本的 Vue 组件,它可以实现视频压缩的功能:
```html
<template>
<div>
<input type="file" @change="handleFileChange">
<button @click="compressVideo">压缩视频</button>
</div>
</template>
<script>
import * as ffmpeg from "ffmpeg.js";
export default {
methods: {
handleFileChange(event) {
this.videoFile = event.target.files[0];
},
async compressVideo() {
const outputFileName = "compressed.mp4";
try {
const video = await this.loadVideo(this.videoFile);
const compressedVideo = await this.compress(video, outputFileName);
this.saveFile(compressedVideo, outputFileName);
} catch (error) {
console.error(error);
}
},
async loadVideo(file) {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = () => {
const video = ffmpeg.FS("writeFile", "input.mp4", new Uint8Array(reader.result));
resolve(video);
};
reader.onerror = (error) => {
reject(error);
};
reader.readAsArrayBuffer(file);
});
},
async compress(video, outputFileName) {
await ffmpeg.load();
await ffmpeg.run("-i", "input.mp4", "-vcodec", "libx264", "-crf", "20", outputFileName);
const compressedVideo = ffmpeg.FS("readFile", outputFileName);
return compressedVideo;
},
saveFile(fileData, fileName) {
const blob = new Blob([fileData.buffer], { type: "video/mp4" });
const url = URL.createObjectURL(blob);
const link = document.createElement("a");
link.href = url;
link.download = fileName;
link.click();
URL.revokeObjectURL(url);
},
},
};
</script>
```
在上面的代码中,我们使用了 `ffmpeg.js` 库来进行视频压缩。首先,我们通过 `FileReader` 对象将用户上传的视频文件读入到 JavaScript 中。然后,我们使用 `ffmpeg.FS()` 方法将视频文件写入到 FFmpeg 文件系统中。接着,我们使用 `ffmpeg.run()` 方法来执行 FFmpeg 命令,将视频文件进行压缩。最后,我们使用 `Blob` 对象和 `a` 标签来将压缩后的视频文件保存到本地。
需要注意的是,`ffmpeg.js` 库比较大,如果需要压缩多个视频文件,建议将该库单独提取出来,并通过 Webpack 等工具进行打包优化。
相关推荐
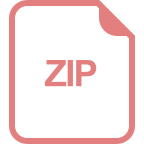
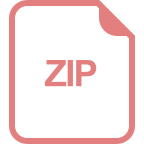












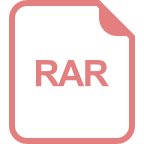
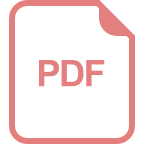