c++如何设置float类型保留位数
时间: 2024-02-26 14:59:22 浏览: 75
在C++中,可以使用std::setprecision()函数来设置float类型的保留位数。例如:
```c++
#include <iostream>
#include <iomanip>
int main() {
float f = 3.1415926;
std::cout << std::fixed << std::setprecision(2) << f << std::endl;
return 0;
}
```
输出结果为:
```
3.14
```
在上面的代码中,使用std::fixed指定输出的浮点数为定点数,并使用std::setprecision(2)设置需要保留的小数点位数为2位。
相关问题
c++float保留几位小数
在C语言中,float类型默认保留6位小数。这意味着当我们将浮点数存储为float类型时,它将保留6位小数,且四舍五入到最接近的值。然而,这并不意味着我们无法控制小数位数的精度。
通过使用printf函数的格式化字符串,我们可以指定要显示的小数位数。例如,如果我们想要将浮点数x保留两位小数并以小数形式打印,我们可以使用以下代码:
```c
printf("%.2f", x);
```
这将会将x打印到控制台,并且只显示两位小数。我们可以修改2为我们想要的任何数值,以适应我们的需求。
另外,我们还可以使用指数表示法来表示float类型的数字。通过使用格式化字符串"%.nf",其中n是我们希望显示的小数位数,我们可以打印出指定精度的指数形式。例如,如果我们想要打印一个浮点数x保留3位小数的指数形式,我们可以使用以下代码:
```c
printf("%.3e", x);
```
这将会将x打印到控制台,并且以指数形式显示,保留三位小数。
总之,在C语言中,float类型默认保留6位小数。但我们可以使用printf函数的格式化字符串来控制显示的小数位数,以满足特定的需求。
c++ float保留两位小数
在C语言中,我们可以使用printf函数将float数据类型保留两位小数输出到屏幕上。具体来说,可以使用格式控制字符串"%.2f",其中百分号后面的".2"表示保留两位小数点,而字母f则表示对应的变量是float类型。
例如,下面的代码可以将一个float类型的变量x保留两位小数输出到屏幕上:
```
float x = 3.1415926;
printf("%.2f", x);
```
这样就能在屏幕上输出"3.14",也就是保留了两位小数的结果。
需要注意的是,保留小数位数只是在输出时起作用,对于实际的float变量本身并不会进行四舍五入或者近似处理,因此我们只能通过输出结果来进行小数位的控制。另外,如果使用其他的数据类型,如double或者long double,也可以通过类似的方式进行小数位的控制。
相关推荐
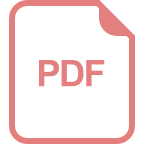
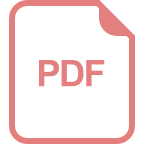
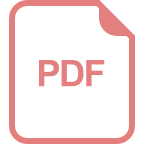












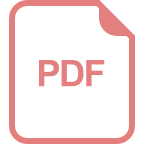