java使用HttpClient传输json格式的参数
时间: 2023-11-21 09:05:16 浏览: 92
可以使用HttpClient的HttpPost方法来传输json格式的参数,具体步骤如下:
1. 创建HttpClient对象:
```
CloseableHttpClient httpClient = HttpClients.createDefault();
```
2. 创建HttpPost对象:
```
HttpPost httpPost = new HttpPost(url);
```
其中,url是接收请求的API地址。
3. 设置请求头:
```
httpPost.setHeader("Content-Type", "application/json");
```
4. 创建请求参数:
```
JSONObject json = new JSONObject();
json.put("param1", "value1");
json.put("param2", "value2");
StringEntity entity = new StringEntity(json.toString(), "UTF-8");
```
其中,param1和param2是接口需要的参数名,value1和value2是参数的具体值。StringEntity将json对象转换为字符串形式,并设置字符编码为UTF-8。
5. 设置请求参数:
```
httpPost.setEntity(entity);
```
6. 发送请求并获取响应:
```
CloseableHttpResponse response = httpClient.execute(httpPost);
```
7. 处理响应结果:
```
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity, "UTF-8");
```
其中,result即为接口返回的结果,可以根据实际需求进行处理。
完整代码示例:
```
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
httpPost.setHeader("Content-Type", "application/json");
JSONObject json = new JSONObject();
json.put("param1", "value1");
json.put("param2", "value2");
StringEntity entity = new StringEntity(json.toString(), "UTF-8");
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity, "UTF-8");
```
阅读全文
相关推荐
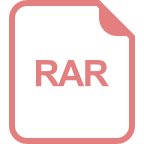
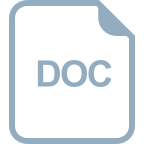
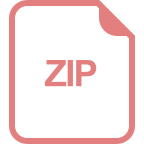
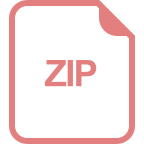
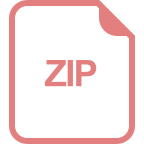
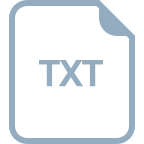
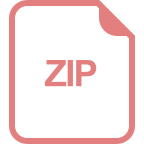
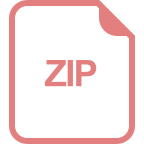
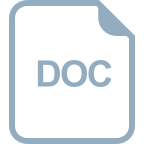
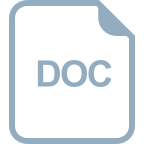




