在c++中使用switch case判断字符串,除了上面的用法外,还有其他方法吗?
时间: 2024-02-23 16:01:57 浏览: 135
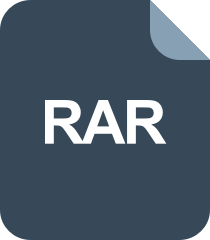
simple_message_handler.rar_C++ 消息分发_If...
除了上面提到的通过字符串的哈希值来实现类似于字符串的 switch case 判断的方法外,还有另外两种常用的方法。
第一种方法是使用 if else 语句进行字符串的判断。代码如下:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "hello";
if (str == "hello") {
std::cout << "Hello World!" << std::endl;
} else if (str == "world") {
std::cout << "Hello World!" << std::endl;
} else {
std::cout << "Unknown string." << std::endl;
}
return 0;
}
```
这种方法简单易懂,但是当需要判断的字符串较多时,代码会比较冗长。
第二种方法是使用 STL 中的 map 容器来保存字符串和对应的处理函数,然后根据字符串在 map 中查找对应的处理函数进行处理。代码如下:
```c++
#include <iostream>
#include <string>
#include <map>
void handle_hello() {
std::cout << "Hello World!" << std::endl;
}
void handle_world() {
std::cout << "Hello World!" << std::endl;
}
int main() {
std::map<std::string, void(*)()> handlers = {
{"hello", handle_hello},
{"world", handle_world}
};
std::string str = "hello";
if (handlers.count(str) > 0) {
handlers[str]();
} else {
std::cout << "Unknown string." << std::endl;
}
return 0;
}
```
这种方法的优点是可以将不同的处理函数分离出来,使代码更加清晰,但是需要额外的函数和 map 容器来管理处理函数,对于简单的应用场景可能会显得过于复杂。
阅读全文
相关推荐
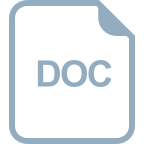
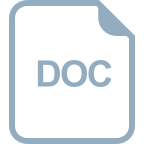


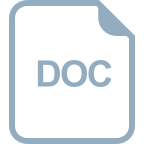
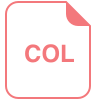
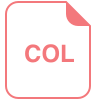




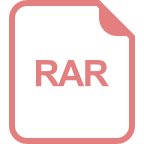
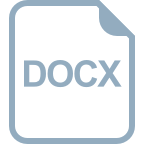
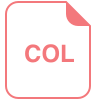
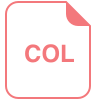


