装饰者模式实例
时间: 2023-06-29 12:15:31 浏览: 66
装饰者模式(Decorator Pattern)是一种结构型设计模式,它允许你动态地将新功能添加到对象中,通过将对象包装在具有新行为的装饰器对象中来实现。
下面是一个示例,使用装饰者模式来实现一个简单的咖啡店程序。首先,我们定义一个抽象基类 `Beverage`,它有一个 `getDescription()` 方法和一个 `cost()` 方法,分别用于描述饮料和计算饮料价格。
```python
from abc import ABC, abstractmethod
class Beverage(ABC):
@abstractmethod
def getDescription(self):
pass
@abstractmethod
def cost(self):
pass
```
然后,我们定义一个具体的饮料类 `Espresso`,它继承自 `Beverage`,实现了 `getDescription()` 和 `cost()` 方法。
```python
class Espresso(Beverage):
def getDescription(self):
return "Espresso"
def cost(self):
return 1.99
```
接下来,我们创建一个装饰器类 `CondimentDecorator`,它也继承自 `Beverage`,并且与 `Beverage` 具有相同的方法。它有一个成员变量 `beverage`,用于存储被装饰的饮料对象。
```python
class CondimentDecorator(Beverage):
def __init__(self, beverage):
self.beverage = beverage
def getDescription(self):
pass
def cost(self):
pass
```
然后,我们定义具体的装饰器类,比如 `Mocha`,它也继承自 `CondimentDecorator`,并且实现了 `getDescription()` 和 `cost()` 方法。
```python
class Mocha(CondimentDecorator):
def getDescription(self):
return self.beverage.getDescription() + ", Mocha"
def cost(self):
return self.beverage.cost() + 0.20
```
最后,我们可以在程序中创建饮料对象,并动态地添加装饰器对象来实现新的功能。
```python
beverage = Espresso()
print(beverage.getDescription(), "$", beverage.cost())
beverage = Mocha(beverage)
print(beverage.getDescription(), "$", beverage.cost())
```
输出结果为:
```
Espresso $ 1.99
Espresso, Mocha $ 2.19
```
这个示例中,我们动态地添加了 `Mocha` 装饰器对象到 `Espresso` 饮料对象中,从而实现了添加 Mocha 的功能。
相关推荐
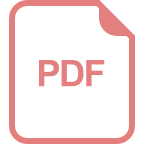
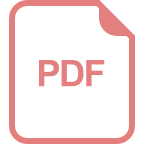
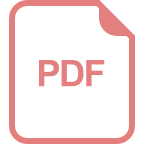













