用C++语言直接写一个代码不要复述文字,要求首先,创建一个基类 Book,包含书的基本信息,如书名、作者、出版社等。这个类可以包含构造函数、析构函数、复制构造函数等成员函数。为了实现多态输出,可以在这个类中编写一个虚函数 print(),用于输出图书的信息。 然后,可以创建一个派生类 EBook,继承自 Book 类,并增加一些新的成员变量,如电子书的文件格式等。这个类中也可以包含构造函数、析构函数等成员函数。 在主函数中,可以创建一个指向 Book 类的指针数组,然后动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。 另外,可以编写一个静态函数 menu(),用于输出操作菜单,让用户选择要进行的操作,例如添加图书、删除图书等。
时间: 2023-02-16 15:21:15 浏览: 107
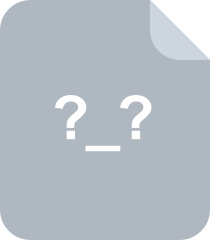
基于c++类的编写
#include <iostream>
#include <string>
class Book {
public:
// 构造函数
Book(std::string name, std::string author, std::string publisher)
: name_(name), author_(author), publisher_(publisher) {}
// 析构函数
~Book() {}
// 复制构造函数
Book(const Book &other)
: name_(other.name_), author_(other.author_), publisher_(other.publisher_) {}
// 虚函数,用于输出图书信息
virtual void print() const {
std::cout << "Book: " << name_ << ", Author: " << author_
<< ", Publisher: " << publisher_ << std::endl;
}
private:
std::string name_;
std::string author_;
std::string publisher_;
};
class EBook : public Book {
public:
// 构造函数
EBook(std::string name, std::string author, std::string publisher, std::string format)
: Book(name, author, publisher), format_(format) {}
// 析构函数
~EBook() {}
// 复制构造函数
EBook(const EBook &other) : Book(other), format_(other.format_) {}
// 覆盖基类的虚函数
void print() const {
std::cout << "EBook: " << name_ << ", Author: " << author_
<< ", Publisher: " << publisher_ << ", Format: " << format_ << std::endl;
}
private:
std::string format_;
};
// 静态函数,输出操作菜单
static void menu() {
std::cout << "Menu:" << std::endl;
std::cout << "1. Add book" << std::endl;
std::cout << "2. Remove book" << std::endl;
std::cout << "3. Print books" << std::endl;
std::cout << "4. Quit" << std::endl;
}
int main() {
// 创建一个指向 Book 类的指针数组
Book *books[10];
// 动态地创建 Book 类的对象和 EBook 类的对象
books[0] = new Book("Book 1", "Author 1", "Publisher 1");
books[1] = new EBook("EBook 1", "Author 2", "Publisher 2", "PDF");
books[2] = new EBook("EBook 2", "Author 3", "Publisher 3", "EPUB");
int choice = 0;
while (choice !=
阅读全文
相关推荐
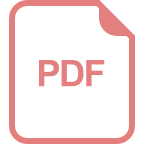
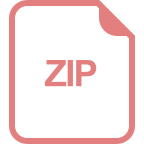



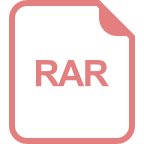
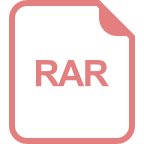
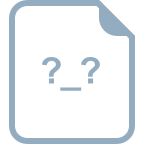
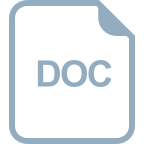
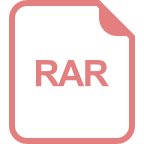
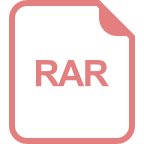
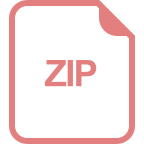
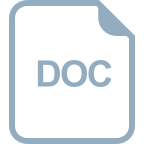
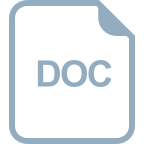
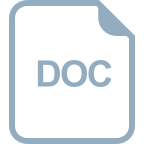
