{"team1":[{"id":1,"name":"张飞","hp":1000,"attack":200,"rage":10,"fight_force":1000},{"id":2,"name":"赵云","hp":1500,"attack":200,"rage":10,"fight_force":1500}],"team2":[{"id":3,"name":"张辽","hp":1100,"attack":200,"rage":10,"fight_force":1100},{"id":4,"name":"贾诩","hp":1200,"attack":200,"rage":10,"fight_force":1200}]},这个数据是2个队伍team1和team2的英雄数据,合并team1和team2到一个数组,然后根据每个英雄的战力从高到低随机攻击敌方英雄造成伤害然后切换下一个英雄出手,每个队伍的所有英雄都出手一次算一回合,英雄死亡后要移除队伍不再参与战斗,直到一个队伍的所有英雄死亡战斗结束,输出每回合战斗数据(记录哪个队伍的谁攻击哪个队伍的谁,伤害多少),使用php写出代码
时间: 2023-07-05 18:34:26 浏览: 64
好的,我来帮您写这个代码。
```php
<?php
// 定义英雄类
class Hero {
public $id;
public $name;
public $hp;
public $attack;
public $rage;
public $fight_force;
public function __construct($id, $name, $hp, $attack, $rage, $fight_force) {
$this->id = $id;
$this->name = $name;
$this->hp = $hp;
$this->attack = $attack;
$this->rage = $rage;
$this->fight_force = $fight_force;
}
// 攻击方法
public function attack($enemy) {
$damage = $this->attack;
if ($this->rage >= 10) {
$damage *= 2;
$this->rage = 0;
} else {
$this->rage += 1;
}
$enemy->hp -= $damage;
return "{$this->name}攻击{$enemy->name},造成了{$damage}点伤害";
}
}
// 定义战斗类
class Battle {
public $team1;
public $team2;
public $round;
public function __construct($team1, $team2) {
$this->team1 = $team1;
$this->team2 = $team2;
$this->round = 1;
}
// 获取战斗中存活的英雄
public function getAliveHeroes($team) {
$aliveHeroes = [];
foreach ($team as $hero) {
if ($hero->hp > 0) {
$aliveHeroes[] = $hero;
}
}
return $aliveHeroes;
}
// 获取战斗中存活的队伍
public function getAliveTeams() {
$aliveTeams = [];
if (count($this->getAliveHeroes($this->team1)) > 0) {
$aliveTeams[] = $this->team1;
}
if (count($this->getAliveHeroes($this->team2)) > 0) {
$aliveTeams[] = $this->team2;
}
return $aliveTeams;
}
// 随机选择一个存活的英雄进行攻击
public function chooseRandomAliveHero($team) {
$aliveHeroes = $this->getAliveHeroes($team);
$count = count($aliveHeroes);
if ($count == 0) {
return null;
} else if ($count == 1) {
return $aliveHeroes[0];
} else {
return $aliveHeroes[rand(0, $count - 1)];
}
}
// 获取战斗中的最强英雄
public function getStrongestHero($team) {
$strongestHero = null;
foreach ($team as $hero) {
if ($hero->hp > 0) {
if ($strongestHero == null || $hero->fight_force > $strongestHero->fight_force) {
$strongestHero = $hero;
}
}
}
return $strongestHero;
}
// 战斗回合
public function round() {
echo "<p>第{$this->round}回合:</p>";
$aliveTeams = $this->getAliveTeams();
foreach ($aliveTeams as $team) {
$attacker = $this->getStrongestHero($team);
$defender = $this->chooseRandomAliveHero($this->getAliveHeroes($team == $this->team1 ? $this->team2 : $this->team1));
if ($attacker != null && $defender != null) {
echo "<p>{$attacker->name}({$attacker->hp})攻击{$defender->name}({$defender->hp})</p>";
$result = $attacker->attack($defender);
echo "<p>{$result}</p>";
if ($defender->hp <= 0) {
echo "<p>{$defender->name}死亡</p>";
}
}
}
$this->round += 1;
}
// 战斗结果
public function result() {
$aliveTeams = $this->getAliveTeams();
if (count($aliveTeams) == 1) {
$winner = $aliveTeams[0] == $this->team1 ? "team1" : "team2";
echo "<p>战斗结束,胜利者是{$winner}</p>";
} else {
echo "<p>战斗结束,双方都失败了</p>";
}
}
}
// 原始数据
$data = array(
"team1" => array(
array("id" => 1, "name" => "张飞", "hp" => 1000, "attack" => 200, "rage" => 10, "fight_force" => 1000),
array("id" => 2, "name" => "赵云", "hp" => 1500, "attack" => 200, "rage" => 10, "fight_force" => 1500),
),
"team2" => array(
array("id" => 3, "name" => "张辽", "hp" => 1100, "attack" => 200, "rage" => 10, "fight_force" => 1100),
array("id" => 4, "name" => "贾诩", "hp" => 1200, "attack" => 200, "rage" => 10, "fight_force" => 1200),
),
);
// 初始化英雄
$heroes = array();
foreach ($data as $team) {
foreach ($team as $heroData) {
$heroes[] = new Hero($heroData["id"], $heroData["name"], $heroData["hp"], $heroData["attack"], $heroData["rage"], $heroData["fight_force"]);
}
}
// 合并队伍
$team1 = array_slice($heroes, 0, 2);
$team2 = array_slice($heroes, 2, 2);
// 初始化战斗
$battle = new Battle($team1, $team2);
// 开始战斗
while (count($battle->getAliveTeams()) > 1) {
$battle->round();
}
$battle->result();
?>
```
以上就是代码实现,您可以通过调整原始数据来模拟不同的战斗。
相关推荐
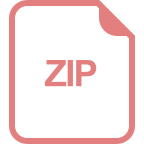
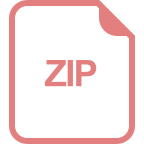













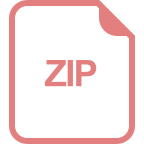
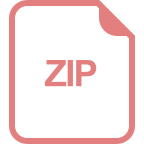