词频统计程序C语言实现
时间: 2024-05-01 08:21:03 浏览: 179
以下是一个简单的词频统计程序的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 50 // 单词最大长度
#define MAX_WORD_NUM 1000 // 最大单词数
typedef struct {
char word[MAX_WORD_LEN]; // 单词
int count; // 出现次数
} WordCount;
int main() {
char text[100000]; // 存储输入文本
WordCount words[MAX_WORD_NUM]; // 存储单词及其出现次数
int wordCount = 0; // 实际单词数
char *token; // 分割后的单词
char delim[] = " ,.?!\"()\n\t"; // 分割符
int i, j, found;
// 读取输入文本
printf("请输入文本:\n");
fgets(text, sizeof(text), stdin);
// 分割字符串,统计单词出现次数
token = strtok(text, delim);
while (token != NULL) {
found = 0;
// 检查该单词是否已经出现过
for (i = 0; i < wordCount; i++) {
if (strcmp(words[i].word, token) == 0) {
words[i].count++;
found = 1;
break;
}
}
// 如果单词未出现过,则加入单词列表
if (!found) {
if (wordCount >= MAX_WORD_NUM) {
printf("单词数超过最大值!\n");
break;
}
strcpy(words[wordCount].word, token);
words[wordCount].count = 1;
wordCount++;
}
token = strtok(NULL, delim);
}
// 按出现次数从大到小排序
for (i = 0; i < wordCount-1; i++) {
for (j = i+1; j < wordCount; j++) {
if (words[i].count < words[j].count) {
WordCount temp = words[i];
words[i] = words[j];
words[j] = temp;
}
}
}
// 输出结果
printf("单词\t\t出现次数\n");
for (i = 0; i < wordCount; i++) {
printf("%-15s%d\n", words[i].word, words[i].count);
}
return 0;
}
```
该程序首先读取用户输入的文本,然后使用strtok()函数分割文本,提取出每个单词。对于每个单词,程序检查其是否已经出现过。如果出现过,则将该单词出现次数加1;如果未出现过,则将该单词加入单词列表,并将其出现次数初始化为1。最后,程序按照单词出现次数从大到小排序,并输出结果。
阅读全文
相关推荐
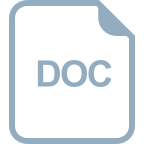
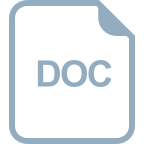
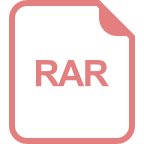
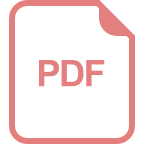
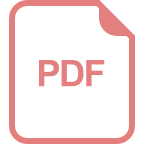
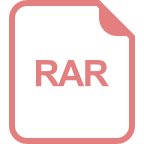
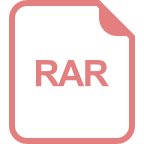
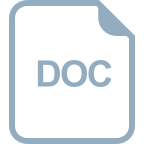
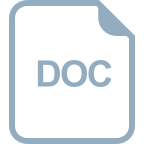
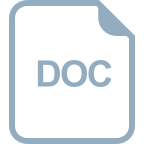
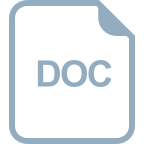
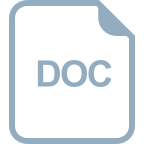



