python实现自适应参数的遗传算法,将步骤用函数实现,给出实现代码
时间: 2024-03-14 11:42:45 浏览: 27
以下是一个将遗传算法步骤封装成函数的 Python 实现,同时使用了自适应参数的方法:
```python
import random
import math
# 定义适应度函数
def fitness(x):
return x * math.sin(10 * math.pi * x) + 2.0
# 定义选择操作
def selection(population, fitness_list):
pop_size = len(population)
sum_fitness = sum(fitness_list)
probability = [fitness_list[j] / sum_fitness for j in range(pop_size)]
cumulative_probability = [sum(probability[:k+1]) for k in range(pop_size)]
new_population = []
for j in range(pop_size):
r = random.random()
for k in range(pop_size):
if r <= cumulative_probability[k]:
new_population.append(population[k])
break
return new_population
# 定义交叉操作
def crossover(population, pc):
chrom_len = len(population[0])
for j in range(0, len(population), 2):
if random.random() < pc:
cpoint = random.randint(1, chrom_len-1)
temp1 = population[j][:cpoint] + population[j+1][cpoint:]
temp2 = population[j+1][:cpoint] + population[j][cpoint:]
population[j], population[j+1] = temp1, temp2
return population
# 定义变异操作
def mutation(population, pm):
chrom_len = len(population[0])
for j in range(len(population)):
if random.random() < pm:
mpoint = random.randint(0, chrom_len-1)
population[j][mpoint] = 1 - population[j][mpoint]
return population
# 定义遗传算法函数
def ga(pop_size=50, chrom_len=20, pc=0.8, pm=0.01, max_iter=100):
# 初始化
population = [[random.randint(0, 1) for _ in range(chrom_len)] for _ in range(pop_size)]
fitness_list = [fitness(int(''.join(map(str, chromosome)), 2)) for chromosome in population]
best_fitness = -9999
best_chromosome = []
# 进化
for i in range(max_iter):
# 选择
new_population = selection(population, fitness_list)
# 交叉
population = crossover(new_population, pc)
# 变异
population = mutation(population, pm)
# 计算适应度
fitness_list = [fitness(int(''.join(map(str, chromosome)), 2)) for chromosome in population]
# 更新参数
if max(fitness_list) > best_fitness:
best_fitness = max(fitness_list)
best_chromosome = population[fitness_list.index(best_fitness)]
pc = min(pc + 0.1, 0.95)
pm = max(pm - 0.01, 0.01)
else:
pc = max(pc - 0.1, 0.2)
pm = min(pm + 0.01, 0.5)
return best_fitness, best_chromosome
# 测试
print(ga())
```
在这个实现中,我们将选择、交叉和变异操作分别封装成了三个函数 `selection`、`crossover` 和 `mutation`。在 `ga` 函数中,我们初始化了一些参数,并随机生成了一个初始种群。然后,我们进行 `max_iter` 次迭代,每次迭代分别进行选择、交叉和变异操作,并计算每个个体的适应度。在每次迭代中,我们还会更新交叉和变异概率 `pc` 和 `pm`,以使算法更加自适应。最后,算法返回最优个体的适应度和染色体。
需要注意的是,这个实现中的自适应参数方法只是一种简单的示例,实际应用中可能需要更复杂的方法来确定参数的变化。
相关推荐
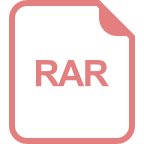
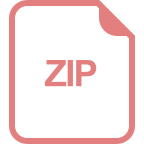














