Java 集成 ONLYOFFICE
时间: 2024-06-08 18:09:20 浏览: 12
ONLYOFFICE 是一个开源的协作办公套件,它可以用于文档处理、电子表格、演示文稿等任务。ONLYOFFICE 支持多种语言,包括 Java。
要集成 ONLYOFFICE 到 Java 项目中,可以使用 ONLYOFFICE 的 REST API。REST API 允许您在 Java 项目中使用 ONLYOFFICE 功能,例如创建、编辑和查看文档。以下是实现这个过程的步骤:
1. 注册 ONLYOFFICE 账户并获取 API 密钥。
2. 在 Java 项目中使用 HTTP 客户端库,例如 Apache HttpClient。
3. 使用 API 密钥调用 ONLYOFFICE REST API,例如通过 POST 请求创建新文档。
4. 使用 ONLYOFFICE 提供的 Web 应用程序作为用户界面,该界面会自动连接到您创建的文档。
以下是一个简单的 Java 示例,用于创建一个新的文档:
```java
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class OnlyOfficeDemo {
public static void main(String[] args) throws Exception {
// 设置 API 密钥和文档名称
String apiKey = "your-api-key";
String documentName = "new-document.docx";
// 创建 HTTP 客户端
HttpClient httpClient = HttpClients.createDefault();
// 创建 POST 请求
HttpPost post = new HttpPost("https://api.onlyoffice.com/editors/create");
// 设置请求头
post.setHeader("Content-Type", "application/json");
// 设置请求体
String requestBody = String.format("{\"key\": \"%s\", \"title\": \"%s\"}", apiKey, documentName);
post.setEntity(new StringEntity(requestBody));
// 发送请求并获取响应
String response = EntityUtils.toString(httpClient.execute(post).getEntity());
// 解析响应并获取文档 URL
String documentUrl = response.substring(response.indexOf("url") + 6, response.indexOf("id") - 3);
// 打开文档
Runtime.getRuntime().exec("rundll32 url.dll,FileProtocolHandler " + documentUrl);
}
}
```
注意,此示例仅用于演示目的。在实际项目中,您需要按照 ONLYOFFICE REST API 文档中的说明进行身份验证、错误处理等操作。
相关推荐
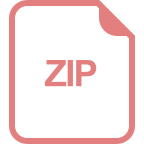
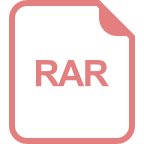
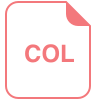
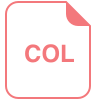
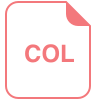
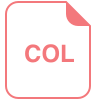
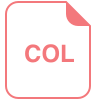









