利用strcpy函数给两个字符数组赋值字符串常量,用strcmp完成两个函数大小 的比较,输出字符串比较的结果。
时间: 2023-07-14 10:13:32 浏览: 44
以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20], str2[20];
// 使用 strcpy 函数赋值字符串常量
strcpy(str1, "Hello");
strcpy(str2, "World");
// 使用 strcmp 函数比较字符串大小
int result = strcmp(str1, str2);
// 输出比较结果
if (result == 0) {
printf("字符串相等\n");
} else if (result < 0) {
printf("str1 小于 str2\n");
} else {
printf("str1 大于 str2\n");
}
return 0;
}
```
输出结果为:
```
str1 小于 str2
```
相关问题
利用strcpy函数给两个字符数组赋值字符串常量,用strcmp完成两个函数大小的比较,输出字符串比较的结果。
下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20], str2[20];
strcpy(str1, "hello");
strcpy(str2, "world");
int cmp = strcmp(str1, str2);
if (cmp < 0) {
printf("%s is less than %s\n", str1, str2);
} else if (cmp > 0) {
printf("%s is greater than %s\n", str1, str2);
} else {
printf("%s and %s are equal\n", str1, str2);
}
return 0;
}
```
运行结果:
```
hello is less than world
```
用strcpy给两个数组赋值字符串常量,用strcmp比较两个函数大小
### 回答1:
这里有一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20], str2[20];
strcpy(str1, "Hello world");
strcpy(str2, "Hello");
int result = strcmp(str1, str2);
if (result == 0) {
printf("两个字符串相等\n");
} else if (result < 0) {
printf("str1 小于 str2\n");
} else {
printf("str1 大于 str2\n");
}
return 0;
}
```
在这个示例中,我们使用了`strcpy`函数将字符串常量赋值给两个字符数组`str1`和`str2`。然后,我们使用`strcmp`比较两个字符串的大小,并将结果存储在一个整数变量`result`中。最后,我们根据`result`的值输出相应的结果。
注意,`strcmp`函数会比较两个字符串的ASCII码值,如果两个字符串相等,则返回0;如果第一个字符串小于第二个字符串,则返回负数;如果第一个字符串大于第二个字符串,则返回正数。
### 回答2:
使用strcpy函数可以将一个字符串常量赋值给一个字符数组。下面给出一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[20];
char str2[20];
// 使用strcpy将字符串常量赋值给两个数组
strcpy(str1, "Hello");
strcpy(str2, "World");
// 使用strcmp比较两个字符串
int result = strcmp(str1, str2);
if (result < 0) {
printf("str1小于str2\n");
} else if (result > 0) {
printf("str1大于str2\n");
} else {
printf("str1等于str2\n");
}
return 0;
}
```
在这个示例中,我们创建了两个字符数组str1和str2,并使用strcpy函数将"Hello"和"World"这两个字符串常量赋值给数组。然后,我们使用strcmp函数来比较这两个字符串,将比较结果存储在result变量中。最后,我们根据比较结果输出相应的提示信息。
需要注意的是,strcmp函数返回的结果是一个整数,如果返回值小于0,则表示第一个字符串小于第二个字符串;如果返回值大于0,则表示第一个字符串大于第二个字符串;如果返回值等于0,则表示两个字符串相等。在上述代码中,我们根据result的值输出相应的比较结果。
### 回答3:
可以使用strcpy函数将一个字符串常量赋值给一个数组,然后再使用strcmp函数比较两个数组的大小。
例如,假设有两个数组str1和str2,我们可以使用strcpy函数将一个字符串常量赋值给这两个数组:
```
#include <stdio.h>
#include <string.h>
int main() {
char str1[20];
char str2[20];
// 使用strcpy将字符串常量赋值给str1和str2
strcpy(str1, "Hello, world!");
strcpy(str2, "Hello, China!");
// 使用strcmp比较str1和str2
int result = strcmp(str1, str2);
if (result > 0) {
printf("str1大于str2\n");
} else if (result < 0) {
printf("str1小于str2\n");
} else {
printf("str1等于str2\n");
}
return 0;
}
```
在上面的代码中,我们使用strcpy函数将字符串常量"Hello, world!"和"Hello, China!"分别赋值给了str1和str2数组,并且使用strcmp函数比较了这两个数组的大小。根据strcmp函数的返回值,我们可以判断出str1和str2的大小关系。
该程序输出的结果是"str1大于str2",因为在ASCII码中,字母'l'的值大于字母'C'的值。
总结:可以使用strcpy函数实现将字符串常量赋值给数组,使用strcmp函数比较两个数组的大小,通过strcmp函数的返回值来判断它们的大小关系。
相关推荐
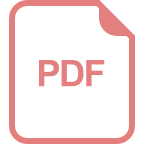
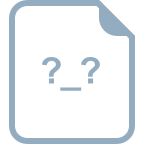
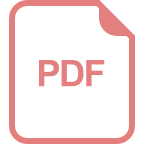
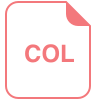
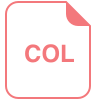
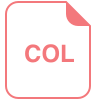









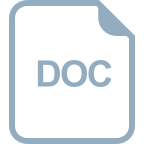