通过dlib人脸关键点实现嘴巴和眼睛实例分割的代码
时间: 2024-02-25 17:57:25 浏览: 100
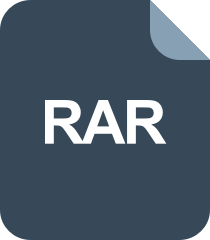
dlib人脸关键点模型
这是一个比较复杂的问题,需要用到多个库和算法。以下是一个基于dlib库和OpenCV库实现嘴巴和眼睛实例分割的示例代码:
```python
import dlib
import cv2
import numpy as np
# 加载dlib的人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载OpenCV的图像和深度学习模型
image = cv2.imread("image.jpg")
net = cv2.dnn.readNetFromCaffe("deploy.prototxt.txt", "res10_300x300_ssd_iter_140000.caffemodel")
# 使用dlib检测人脸,并提取关键点
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
rects = detector(gray, 1)
for (i, rect) in enumerate(rects):
shape = predictor(gray, rect)
shape = np.array([[p.x, p.y] for p in shape.parts()])
# 提取嘴巴区域
mouth_points = shape[48:68]
mouth_mask = np.zeros(image.shape[:2], dtype=np.uint8)
cv2.drawContours(mouth_mask, [mouth_points], -1, 255, -1)
# 提取眼睛区域
left_eye_points = shape[36:42]
right_eye_points = shape[42:48]
eyes_mask = np.zeros(image.shape[:2], dtype=np.uint8)
cv2.drawContours(eyes_mask, [left_eye_points], -1, 255, -1)
cv2.drawContours(eyes_mask, [right_eye_points], -1, 255, -1)
# 使用深度学习模型检测人脸
(h, w) = image.shape[:2]
blob = cv2.dnn.blobFromImage(cv2.resize(image, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
net.setInput(blob)
detections = net.forward()
# 遍历检测结果,提取人脸区域
for j in range(0, detections.shape[2]):
confidence = detections[0, 0, j, 2]
if confidence < 0.5:
continue
box = detections[0, 0, j, 3:7] * np.array([w, h, w, h])
(startX, startY, endX, endY) = box.astype("int")
# 只保留人脸区域内的眼睛和嘴巴区域
eyes_mask = eyes_mask[startY:endY, startX:endX]
mouth_mask = mouth_mask[startY:endY, startX:endX]
# 对眼睛和嘴巴区域进行实例分割
eyes_output = cv2.bitwise_and(image[startY:endY, startX:endX], image[startY:endY, startX:endX], mask=eyes_mask)
mouth_output = cv2.bitwise_and(image[startY:endY, startX:endX], image[startY:endY, startX:endX], mask=mouth_mask)
# 显示结果
cv2.imshow("Eyes Output", eyes_output)
cv2.imshow("Mouth Output", mouth_output)
cv2.waitKey(0)
```
需要注意的是,这个示例代码只能检测一张人脸,并提取其中的嘴巴和眼睛区域进行实例分割。如果需要处理多张人脸的情况,需要对代码进行修改。
阅读全文
相关推荐
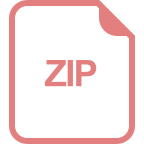







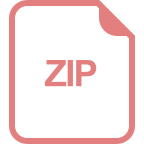
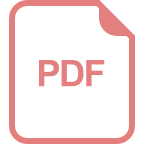
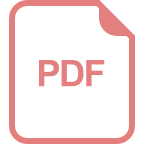
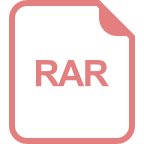
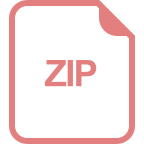
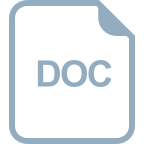


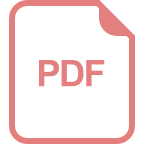