用springboot框架写一个购物车实现
时间: 2024-03-13 20:37:57 浏览: 21
由于购物车的实现需要前端页面的交互,后端只提供数据接口,所以本文只提供后端代码实现。
1. 创建商品实体类
首先需要定义商品实体类,包含商品ID,名称,价格等信息。
```java
@Entity
@Table(name = "product")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String name;
@Column(nullable = false)
private double price;
public Product() {
}
public Product(String name, double price) {
this.name = name;
this.price = price;
}
// getter and setter methods
}
```
2. 创建购物车实体类
购物车实体类包含购物车ID,用户ID,购物车内商品列表等信息。
```java
@Entity
@Table(name = "cart")
public class Cart {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private Long userId;
@OneToMany(mappedBy = "cart", cascade = CascadeType.ALL, orphanRemoval = true)
private List<CartItem> cartItems = new ArrayList<>();
public Cart() {
}
public Cart(Long userId) {
this.userId = userId;
}
// getter and setter methods
}
```
3. 创建购物车商品实体类
购物车商品实体类包含购物车商品ID,购物车ID,商品ID,商品数量等信息。
```java
@Entity
@Table(name = "cart_item")
public class CartItem {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "cart_id")
private Cart cart;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "product_id")
private Product product;
@Column(nullable = false)
private int quantity;
public CartItem() {
}
public CartItem(Cart cart, Product product, int quantity) {
this.cart = cart;
this.product = product;
this.quantity = quantity;
}
// getter and setter methods
}
```
4. 创建商品Repository
使用Spring Data JPA创建商品Repository,在这里提供了根据商品ID查询商品的方法。
```java
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
Optional<Product> findById(Long id);
}
```
5. 创建购物车Repository
使用Spring Data JPA创建购物车Repository,提供根据用户ID查询购物车的方法。
```java
@Repository
public interface CartRepository extends JpaRepository<Cart, Long> {
Optional<Cart> findByUserId(Long userId);
}
```
6. 创建购物车服务类
购物车服务类定义了添加商品到购物车,从购物车中移除商品以及获取购物车中所有商品的方法。
```java
@Service
public class CartService {
@Autowired
private CartRepository cartRepository;
@Autowired
private ProductRepository productRepository;
public void addItemToCart(Long userId, Long productId, int quantity) {
Optional<Cart> optionalCart = cartRepository.findByUserId(userId);
Cart cart = optionalCart.orElse(new Cart(userId));
Optional<Product> optionalProduct = productRepository.findById(productId);
Product product = optionalProduct.orElseThrow(() -> new RuntimeException("Product not found"));
List<CartItem> cartItems = cart.getCartItems();
boolean itemFound = false;
for (CartItem cartItem : cartItems) {
if (cartItem.getProduct().getId().equals(productId)) {
cartItem.setQuantity(cartItem.getQuantity() + quantity);
itemFound = true;
break;
}
}
if (!itemFound) {
CartItem cartItem = new CartItem(cart, product, quantity);
cartItems.add(cartItem);
}
cartRepository.save(cart);
}
public void removeItemFromCart(Long userId, Long productId) {
Optional<Cart> optionalCart = cartRepository.findByUserId(userId);
Cart cart = optionalCart.orElseThrow(() -> new RuntimeException("Cart not found"));
List<CartItem> cartItems = cart.getCartItems();
CartItem itemToRemove = null;
for (CartItem cartItem : cartItems) {
if (cartItem.getProduct().getId().equals(productId)) {
itemToRemove = cartItem;
break;
}
}
if (itemToRemove != null) {
cartItems.remove(itemToRemove);
cartRepository.save(cart);
}
}
public List<CartItem> getCartItems(Long userId) {
Optional<Cart> optionalCart = cartRepository.findByUserId(userId);
Cart cart = optionalCart.orElseThrow(() -> new RuntimeException("Cart not found"));
return cart.getCartItems();
}
}
```
7. 创建控制器
定义了添加商品到购物车,从购物车中移除商品以及获取购物车中所有商品的API接口。
```java
@RestController
@RequestMapping("/cart")
public class CartController {
@Autowired
private CartService cartService;
@PostMapping("/add")
public void addItemToCart(@RequestParam("userId") Long userId,
@RequestParam("productId") Long productId,
@RequestParam("quantity") int quantity) {
cartService.addItemToCart(userId, productId, quantity);
}
@PostMapping("/remove")
public void removeItemFromCart(@RequestParam("userId") Long userId,
@RequestParam("productId") Long productId) {
cartService.removeItemFromCart(userId, productId);
}
@GetMapping("/items")
public List<CartItem> getCartItems(@RequestParam("userId") Long userId) {
return cartService.getCartItems(userId);
}
}
```
这样就实现了购物车的基本功能,可以通过API接口实现购物车的添加商品,移除商品以及获取购物车所有商品的功能。
相关推荐
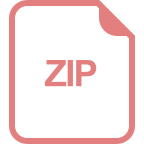
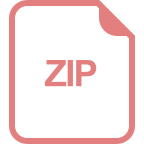
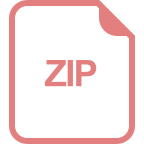
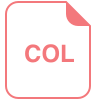
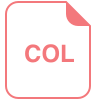
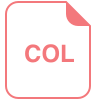
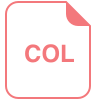










