okhttp android socket 框架实例 带发送消息的方法
时间: 2023-12-28 14:05:46 浏览: 161
以下是一个使用 OkHttp 实现 Socket 通信的示例代码:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.Socket;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import okhttp3.ResponseBody;
import okio.BufferedSink;
public class SocketClient {
private static final String HOST = "example.com"; // 服务器地址
private static final int PORT = 12345; // 服务器端口号
private static final MediaType MEDIA_TYPE_PLAIN_TEXT = MediaType.parse("text/plain");
private OkHttpClient client;
private Socket socket;
private BufferedSink sink;
public void connect() throws IOException {
client = new OkHttpClient();
socket = new Socket();
socket.connect(new InetSocketAddress(HOST, PORT), 5000); // 连接超时时间为 5 秒
sink = Okio.buffer(Okio.sink(socket));
}
public void disconnect() throws IOException {
socket.close();
}
public void send(String message) throws IOException {
sink.writeUtf8(message);
sink.flush();
}
public String receive() throws IOException {
Request request = new Request.Builder()
.url("http://" + HOST + ":" + PORT)
.post(RequestBody.create(MEDIA_TYPE_PLAIN_TEXT, ""))
.build();
Response response = client.newCall(request).execute();
ResponseBody body = response.body();
if (body == null) {
return null;
}
return body.string();
}
}
```
在上面的代码中,我们使用 OkHttp 的 `OkHttpClient` 类来发送 HTTP POST 请求,以接收服务器发送的消息。`send` 方法使用 Okio 的 `BufferedSink` 类将消息写入 Socket 连接。使用时需要注意在合适的时机调用 `connect` 方法连接服务器,并在不需要连接时调用 `disconnect` 方法断开连接。
阅读全文
相关推荐
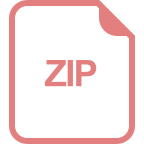

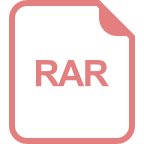
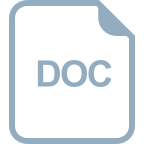
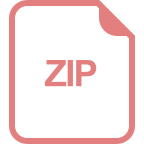
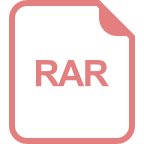
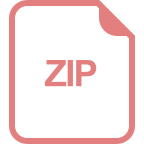
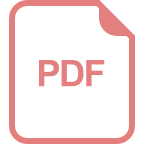
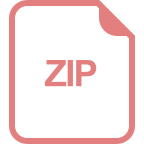
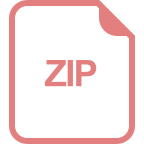
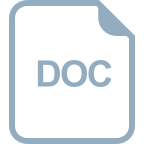
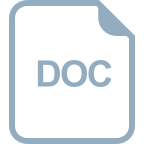
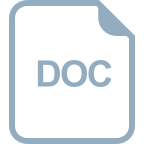
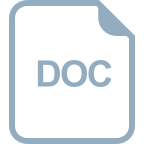
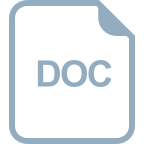
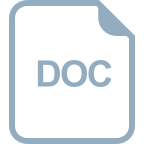
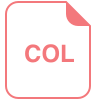