Java代码创建Postgresql表
时间: 2023-07-16 20:16:53 浏览: 162
以下是使用Java代码创建PostgreSQL表的示例:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.Statement;
import java.sql.SQLException;
public class CreateTable {
public static void main(String[] args) {
Connection connection = null;
Statement statement = null;
try {
// 注册驱动
Class.forName("org.postgresql.Driver");
// 创建连接
connection = DriverManager.getConnection("jdbc:postgresql://localhost:5432/mydatabase", "myuser", "mypassword");
// 创建语句
statement = connection.createStatement();
// 创建表
String createTableSQL = "CREATE TABLE employees ("
+ "id serial primary key,"
+ "name varchar(100),"
+ "age int,"
+ "salary numeric(10,2)"
+ ")";
statement.execute(createTableSQL);
System.out.println("Table created successfully!");
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
try {
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例中,我们使用JDBC连接到PostgreSQL数据库并创建一个名为"employees"的表。该表包含四个列:id、name、age和salary。
请注意,在使用Java代码创建数据库表时,您需要确保在创建表之前已经创建了相应的数据库。同时,还需要注意数据库连接的用户名和密码。
阅读全文
相关推荐
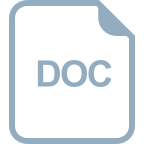
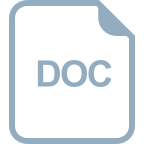
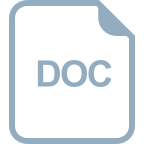
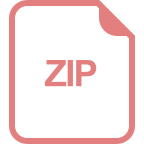
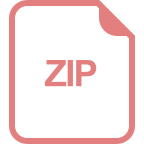
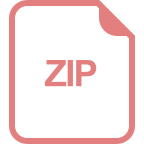
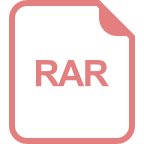
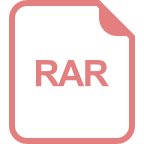
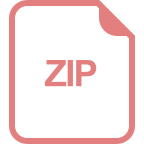
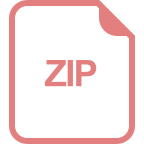
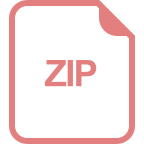
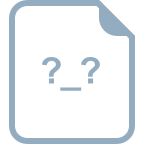
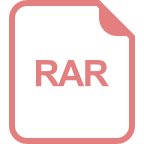
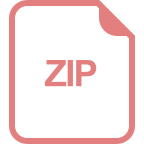
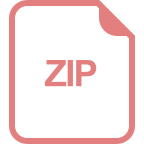
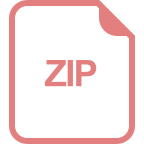
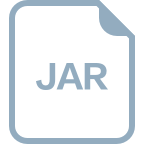
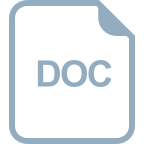
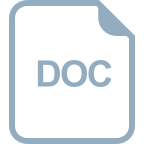