编写程序,读取存储若干数字的 文件,对其中的数字进行排序后 输出。Python
时间: 2023-11-27 11:49:12 浏览: 159
假设文件每行只有一个数字,可以使用以下代码实现:
```python
# 读取文件并存储到列表中
with open('filename.txt', 'r') as file:
numbers = [int(line.strip()) for line in file]
# 对数字进行排序
numbers.sort()
# 输出排序后的数字
for num in numbers:
print(num)
```
其中,`with open('filename.txt', 'r') as file` 语句打开文件,并将其存储为 `file` 对象。`for line in file` 循环遍历文件的每一行,`line.strip()` 去除每行字符串首尾的空白字符,`int()` 将字符串转换为整数类型,并将所有数字存储到 `numbers` 列表中。
`numbers.sort()` 对列表中的数字进行排序,默认为升序排序。
最后使用循环输出排序后的数字。
相关问题
使用python编写程序 读取存储若干数字的文件,对其中的数字进行排序后输出。
好的,以下是一个使用Python编写的示例程序,可以读取存储数字的文件,对数字进行排序并输出:
```python
def read_numbers_from_file(file_path):
numbers = []
with open(file_path, 'r') as file:
for line in file:
number = int(line.strip())
numbers.append(number)
return numbers
def sort_numbers(numbers):
numbers.sort()
def write_numbers_to_file(file_path, numbers):
with open(file_path, 'w') as file:
for number in numbers:
file.write(str(number) + '\n')
# 读取文件中的数字
file_path = 'numbers.txt' # 替换为你的文件路径
numbers = read_numbers_from_file(file_path)
# 对数字进行排序
sort_numbers(numbers)
# 输出排序后的数字
for number in numbers:
print(number)
# 将排序后的数字写入文件
output_file_path = 'sorted_numbers.txt' # 替换为你的输出文件路径
write_numbers_to_file(output_file_path, numbers)
```
请将上述代码保存为一个Python文件(例如:`sort_numbers.py`),并将待排序的数字存储在名为 `numbers.txt` 的文件中。然后运行该程序即可。排序后的结果将会在控制台输出,并且会生成一个名为 `sorted_numbers.txt` 的文件,其中包含了排序后的数字。请确保替换文件路径为你实际使用的路径。
编写程序,读取存储若干数字的文件,对其中的数字进行排序后输出
### 回答1:
好的,以下是编写程序读取存储若干数字的文件,对其中的数字进行排序后输出的代码示例:
```python
# 打开文件并读取数字
with open('numbers.txt', 'r') as f:
numbers = [int(num) for num in f.read().split()]
# 对数字进行排序
numbers.sort()
# 输出排序后的数字
print(numbers)
```
其中,`numbers.txt`是存储数字的文件名,程序会读取文件中的数字并存储到列表`numbers`中,然后使用`sort()`方法对数字进行排序,最后输出排序后的数字列表。
### 回答2:
编写程序读取存储若干数字的文件,是一项常见的任务。一般来说,对数字进行排序的算法有很多,比如冒泡排序、快速排序、选择排序等等,针对不同场景和需求,可以选用不同的算法。下面我将按照一个基础的思路,来介绍一种简单的读取文件、排序和输出的程序实现。
首先,需要打开保存数字的文件,读取其中的数字,并将它们存储到一个数组中。可以使用Python语言来实现这个过程,具体代码如下:
```
with open('data.txt', 'r') as f:
nums = []
for line in f.readlines():
nums.append(int(line.strip()))
```
上述代码中,文件名为data.txt,使用open函数打开该文件,并使用readlines方法逐行读取其中的数字,然后将每个数字转换为整数类型后,添加到一个新的列表nums中。读取完毕后,可以关闭文件,继续进行排序过程。
排序的核心是算法,这里选择使用Python内置的sort函数,它可以快速地对列表元素进行排序。这里给出一个简单的冒泡排序实现,代码如下:
```
for i in range(len(nums)-1):
for j in range(len(nums)-i-1):
if nums[j] > nums[j+1]:
nums[j], nums[j+1] = nums[j+1], nums[j]
```
上述代码中,通过双重循环来实现冒泡排序,外层循环控制排序的次数,内层循环用来比较相邻元素的大小,并交换它们的位置。排序完成后,可以使用print函数在控制台上输出排序后的结果,代码如下:
```
print(nums)
```
上述代码将nums数组输出到控制台,完成了对存储若干数字的文件进行排序后输出的任务。
总之,编写程序读取存储若干数字的文件,并对其中的数字进行排序,可以使用Python语言和常见的排序算法,通过文件读取、数组排序和控制台输出等步骤来实现。需要注意的是,具体的实现方式需要根据实际场景和需求来选择。
### 回答3:
编写程序实现读取存储若干数字的文件并对其中的数字进行排序后输出的过程,需要经历以下几个主要步骤:
1. 读取文件中的数字
首先需要用程序读取文件中存储的数字,可以使用C++中的文件流操作,通过打开文件、读取文件中的数字,然后关闭文件的方式进行读取。程序需要保证文件格式正确,文件中每行只能有一个数字。
2. 排序
读取文件中的数字后,需要将这些数字进行排序。可以选择合适的排序算法,如快速排序、冒泡排序、选择排序等。在程序中使用具体的排序算法,对读取到的数字进行排序。
3. 输出结果
对文件中的数字进行排序后,需要将结果输出。可以选择将排序结果打印在控制台上,或者将结果写入一个新建的文本文件中。最后,程序应该关闭文件和释放内存空间,以保证程序的稳定性。
对于具体的实现过程,需要根据所使用的编程语言和排序算法进行具体调整,但以上3个步骤是编写这样一个程序必不可少的主要步骤,应该注意代码的健壮性和可靠性,以确保程序的正确性和安全性。
相关推荐
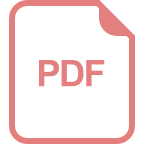












